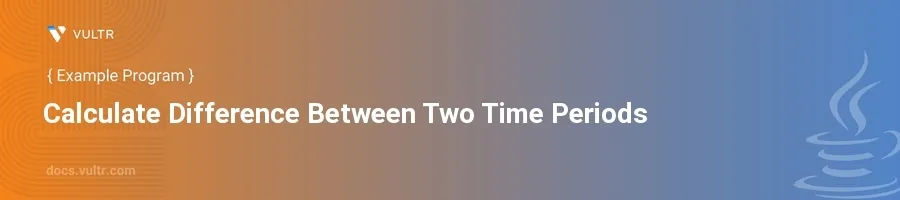
Introduction
Calculating the difference between two time periods in Java is a common task in many software applications, ranging from logging systems to scheduling and time management tools. Correct and precise time calculation ensures accurate data processing and user-friendly interfaces.
In this article, you will learn how to calculate the difference between two time periods using Java. You'll explore various examples showcasing different scenarios, such as the difference between two time instances in hours, minutes, and seconds.
Basic Time Difference Calculation
Setting Up Time Instances
Utilize Java's
LocalTime
class from thejava.time
package to represent time.Create two
LocalTime
instances for the start and end times.javaimport java.time.LocalTime; import java.time.Duration; LocalTime startTime = LocalTime.of(9, 30, 0); // 9:30:00 LocalTime endTime = LocalTime.of(17, 45, 30); // 17:45:30
Here, you create
startTime
andendTime
objects representing 9:30 AM and 5:45 PM respectively.
Calculating Duration Between Times
Use
Duration.between()
to find the difference betweenstartTime
andendTime
.Extract the duration in hours, minutes, and seconds.
javaDuration duration = Duration.between(startTime, endTime); long hours = duration.toHours(); long minutes = duration.toMinutes() % 60; long seconds = duration.getSeconds() % 60;
This block of code calculates the total hours, remaining minutes after hours, and remaining seconds after minutes between the two specified times.
Output the Result
Use
System.out.println
to display the calculated time difference.javaSystem.out.println("Difference: " + hours + " Hours " + minutes + " Minutes " + seconds + " Seconds");
The output will display the total duration between the two time instances in a readable format.
Handling Dates and Times Together
Using LocalDateTime
If dealing with both dates and times, utilize the
LocalDateTime
class.Create
LocalDateTime
instances and follow a similar approach as withLocalTime
.javaimport java.time.LocalDateTime; LocalDateTime startDateTime = LocalDateTime.of(2021, 10, 5, 9, 30, 0); // Oct 5, 2021, 9:30:00 LocalDateTime endDateTime = LocalDateTime.of(2021, 10, 5, 17, 45, 30); // Oct 5, 2021, 17:45:30 Duration duration = Duration.between(startDateTime, endDateTime); System.out.println("Total seconds: " + duration.getSeconds());
This example calculates the total seconds between two
LocalDateTime
instances, which includes the date and the time.
Dealing with Time Zones
Calculating With TimeZone Adjustments
For time-sensitive applications involving multiple time zones, use
ZonedDateTime
.Adjust the time zone using the
ZoneId
to ensure accuracy across different regions.javaimport java.time.ZonedDateTime; import java.time.ZoneId; ZonedDateTime zonedStartDateTime = ZonedDateTime.of(LocalDateTime.of(2021, 10, 5, 9, 30, 0), ZoneId.of("America/New_York")); ZonedDateTime zonedEndDateTime = ZonedDateTime.of(LocalDateTime.of(2021, 10, 5, 17, 45, 30), ZoneId.of("America/Los_Angeles")); Duration duration = Duration.between(zonedStartDateTime, zonedEndDateTime); System.out.println("Total hours difference: " + duration.toHours());
Here, you can see how to handle time differences when start and end times are in different time zones (New York and Los Angeles).
Conclusion
Calculating the difference between two time periods in Java helps in developing time-sensitive applications that require precise scheduling and logging. This can involve anything from simple hour calculations to dealing with complex time zones. With Java's robust java.time
package, perform these operations reliably for applications that need to manage time precisely. By mastering these techniques, you ensure your applications behave consistently and as expected across different contexts and regions.
No comments yet.