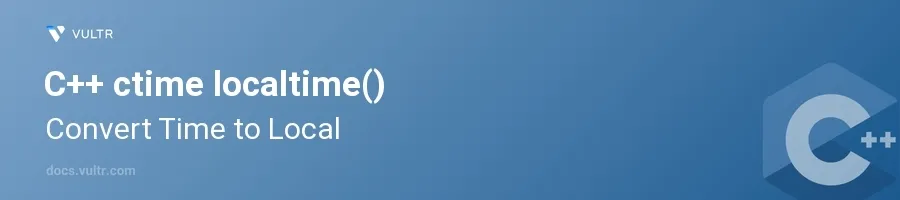
Introduction
The localtime()
function in C++ is part of the <ctime>
header and provides a convenient way to convert a timestamp, represented as a time_t object, into local time. This function is widely used in applications that require time manipulation and display based on the local time zone of the system where the application is running.
In this article, you will learn how to use the localtime()
function in various real-world scenarios. Grasp the essentials of working with time data, converting timestamps to more readable formats, and handling the returned structure for further time-based operations in C++.
Utilizing localtime() in C++
Convert Epoch Time to Local Time
Include the
<ctime>
header in your C++ program to access time manipulation functions.Obtain the current time using
time()
, which provides the time since the UNIX epoch (January 1, 1970).Convert the epoch time to local time using
localtime()
.cpp#include <iostream> #include <ctime> int main() { time_t now = time(0); tm *ltm = localtime(&now); std::cout << "Current local time : " << ltm->tm_hour << ":" << ltm->tm_min << ":" << ltm->tm_sec << std::endl; return 0; }
This code snippet fetches the current time in seconds since the epoch and converts it into local time. It then prints out the local time in hours, minutes, and seconds.
Using localtime() to Format Date and Time
After converting the timestamp to local time using
localtime()
, extract various components like day, month, year, etc.Format these components into a more human-readable date-time format.
cpp#include <iostream> #include <ctime> int main() { time_t now = time(0); tm *ltm = localtime(&now); std::cout << "Date: "; std::cout << ltm->tm_mday << "-"; std::cout << 1 + ltm->tm_mon << "-"; // Months since January – add 1 for correct month std::cout << 1900 + ltm->tm_year << std::endl; // Years since 1900 – add 1900 for correct year return 0; }
This snippet prints the current date by extracting the day, month, and year from the local time structure. Note the adjustment for months and years as
tm_mon
andtm_year
are offsets from January and 1900, respectively.
Handling Time Zones with localtime()
Understand that
localtime()
automatically adjusts the time according to the system's local time zone.Consider time zone implications when deploying applications across different geographical locations.
- Time zone handling is critical for applications that operate over multiple regions.
localtime()
uses the time zone settings of the host machine, which simplifies development but requires awareness when handling globally distributed systems.
Conclusion
The localtime()
function from the C++ <ctime>
library offers a straightforward method for converting epoch time into local time, making it simpler to work with time data in human-readable formats. By mastering localtime()
, you can effectively handle time conversion and presentation, enhancing the functionality and user-friendliness of your applications. Implement the techniques discussed here to manage time data efficiently and accurately in your C++ projects.
No comments yet.