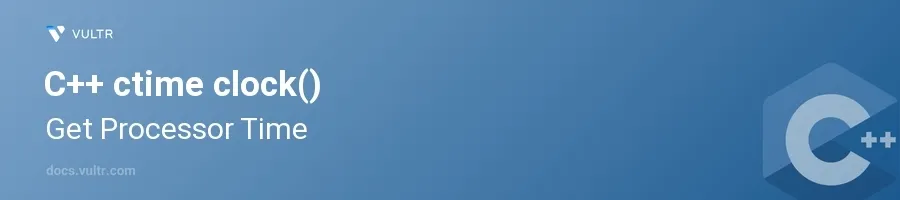
Introduction
In C++, clock()
from the <ctime>
library is a standard function used to measure processor time consumed by the program's execution. This can prove particularly useful for benchmarking purposes or to optimize the performance of your code by measuring the time taken by specific sections of your code to execute.
In this article, you will learn how to utilize the clock()
function effectively to gather processor times for different code sections. Also discussed are common pitfalls and pointers on how to interpret the results accurately for improved code performance assessment.
Basic Usage of clock()
Measure Complete Program Execution Time
Include the
<ctime>
library and start the clock at the beginning of themain()
function.Capture the clock value at the end of your program and calculate the elapsed time.
cpp#include <iostream> #include <ctime> int main() { clock_t start = clock(); // Program code here clock_t end = clock(); double elapsed = double(end - start) / CLOCKS_PER_SEC; std::cout << "Elapsed time: " << elapsed << " seconds." << std::endl; return 0; }
This code demonstrates the basic setup for using
clock()
. The elapsed time is calculated by subtracting the start time from the end time, then dividing byCLOCKS_PER_SEC
to convert to seconds. The result provides how long the CPU spent executing your program.
Timing a Specific Function or Code Segment
Implement
clock()
to measure time taken by specific functions.Capture the start and end time before and after the function or section of code.
cppvoid functionToTime() { // Function code here } int main() { clock_t start = clock(); functionToTime(); clock_t end = clock(); double elapsed = double(end - start) / CLOCKS_PER_SEC; std::cout << "Function time: " << elapsed << " seconds." << std::endl; return 0; }
This snippet extends the previous example by enclosing a specific function call within the timing checks. Similarly, the elapsed time shows how long that specific function takes to execute.
Interpreting the Results
Understanding clock()
Limitations
Realize
clock()
measures CPU time, not wall-clock time.Remember that I/O operations do not typically consume CPU time.
The
clock()
function measures the amount of processor time the program consumes, which is different from real-time (especially when multitasking or waiting for I/O operations). In multicore systems,clock()
does not account for time spent in parallel execution on different cores.
Using clock()
in Multi-threaded Applications
Acknowledge that
clock()
measures time for each thread separately in many environments.Use thread-specific timing functions or enhancements for more accurate multi-threading evaluations.
Since
clock()
might cumulatively add times from all threads, this can lead to overestimating the time required by parallel programs. For accurate parallel execution timing, consider using platform-specific threading libraries that provide precise timings for individual threads.
Conclusion
The clock()
function in C++ is a powerful tool for measuring processor time used by segments of your code, facilitating performance analysis and optimizations. Proper utilization not only helps in getting a glimpse into the computational cost of functional components but also aids in making informed decisions about code enhancements. Implement the insights and examples provided to ensure your applications are efficient and performant.
No comments yet.