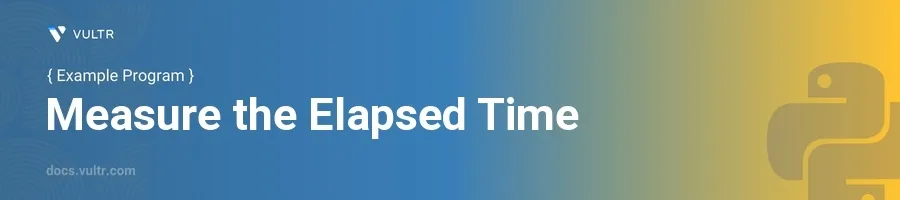
Introduction
Measuring elapsed time in a Python program is crucial for performance analysis, debugging, and optimizing the code. Whether timing how long a function takes to run or determining the duration of a user's session, having an accurate and simple way to measure time is essential for many applications. Python provides several methods and libraries to handle high-resolution timing and profiling effectively.
In this article, you will learn how to measure elapsed time in Python using different methods. Discover how to implement these techniques through simple examples that you can integrate into your Python scripts for performance testing or timing operations.
Using time Module for Elapsed Time
Using time.time()
to Measure Time
Import the
time
module.Record the start time before the block of code you want to time.
Execute the code.
Record the end time after the code execution.
Calculate the elapsed time by subtracting the start time from the end time.
pythonimport time start_time = time.time() # Example: Simulate a code block that takes time, e.g., a sleep for 3 seconds time.sleep(3) end_time = time.time() elapsed_time = end_time - start_time print(f"Elapsed Time: {elapsed_time} seconds")
This example calculates the time taken by
time.sleep(3)
function.time.time()
returns the current time in seconds since the Epoch (January 1, 1970, 00:00:00 (UTC)). The difference betweenend_time
andstart_time
gives the elapsed time in seconds.
Using time.perf_counter()
for High Resolution Time
Import the
time
module.Use
time.perf_counter()
instead oftime.time()
for higher resolution and more precise measurements.Follow the same steps of recording start and end times and calculating the difference.
pythonimport time start = time.perf_counter() # Performing a detailed task time.sleep(2) end = time.perf_counter() elapsed = end - start print(f"High Resolution Elapsed Time: {elapsed} seconds")
time.perf_counter()
provides a higher resolution timer, which is more precise, especially useful for measuring smaller time durations. It also includes time elapsed during sleep and is system-wide.
Using datetime Module for Elapsed Time
Measuring Time with datetime.datetime.now()
Import the
datetime
module.Record the current time using
datetime.datetime.now()
both at the start and after the code execution.Calculate the elapsed time by subtracting the start time from the end time.
pythonfrom datetime import datetime start = datetime.now() # Example operation time.sleep(1) end = datetime.now() elapsed = end - start print(f"Elapsed Time using datetime: {elapsed}")
This snippet makes use of
datetime.now()
which returns the current local date and time. The resultant elapsed time is adatetime.timedelta
object, which provides the difference in a more readable form compared to raw seconds.
Conclusion
Measuring elapsed time in Python can be achieved through various methods, each suitable for different scenarios and precision requirements. Whether you choose to use the time
module with its straightforward functions like time.time()
or time.perf_counter()
, or opt for the datetime
module's datetime.now()
, you have powerful tools at your disposal to monitor the duration of operations within your applications. Implement these strategies to gain insights into performance and optimize your Python programs efficiently.
No comments yet.