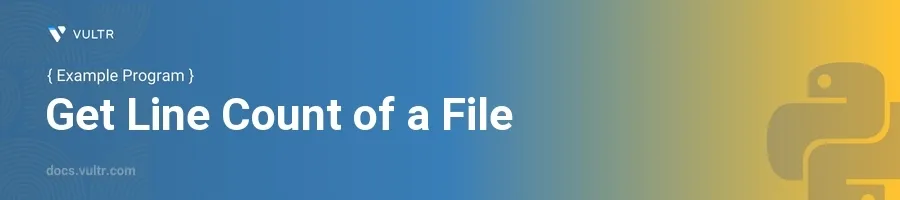
Introduction
Understanding how to count lines in a file is a fundamental skill for many Python developers, especially when dealing with data processing, logging, or file management tasks. Python provides a variety of methods to accomplish line counting in a straightforward manner, often needed for tasks like data analysis or monitoring file changes.
In this article, you will learn how to use Python effectively to count the lines in a file. You'll explore different methods including reading the entire file, iterating through each line, and using libraries that Python offers to handle file operations efficiently.
Counting Lines Using Basic Python
Open and Read File Method
Open the file in read mode.
Read the file content and count the newlines.
pythonwith open('example.txt', 'r') as file: contents = file.read() line_count = contents.count('\n') print("Total lines:", line_count)
This code opens
example.txt
and reads the entire content into thecontents
variable. The.count('\n')
then counts how many newline characters are present, essentially giving the number of lines in the file.
Line Iteration Method
Open the file in read mode.
Iterate over each line in the file and increment a counter.
pythonline_count = 0 with open('example.txt', 'r') as file: for line in file: line_count += 1 print("Total lines:", line_count)
The above method iterates through each line using a
for
loop. For every iteration (a line), the counterline_count
is incremented by one.
Using Python Libraries
Counting Lines with fileinput
Import the
fileinput
module.Use the
fileinput.input()
function to iterate through lines across multiple files.pythonimport fileinput line_count = sum(1 for line in fileinput.input('example.txt')) print("Total lines:", line_count)
fileinput.input()
creates an iterator over the lines of the specified file. Thesum(1 for line in ...)
pattern efficiently counts the lines as it iterates through the file. This method is particularly useful when handling multiple files.
Using pandas
for Large Data Files
Install the
pandas
library if not already installed (pip install pandas
).Import
pandas
and use theread_csv
function.pythonimport pandas as pd df = pd.read_csv('large_data_file.csv') line_count = len(df.index) print("Total lines:", line_count)
In this snippet,
read_csv
is used to load a CSV file into a DataFrame. The number of rowslen(df.index)
in the DataFrame corresponds to the number of lines in the file, excluding the header. This method is ideal for CSV files and also allows manipulation of the data if needed.
Conclusion
Counting lines in a file using Python can be approached through multiple methods each suitable for different scenarios. Whether reading the entire file into memory or processing line by line for larger files, Python's versatile functionalities like fileinput
and libraries like pandas
make it adaptable for both small and large-scale file operations. Apply these methods based on your specific needs to efficiently handle file operations in your projects.
No comments yet.