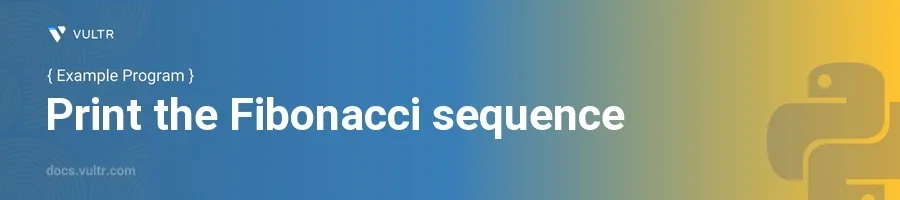
Introduction
The Fibonacci sequence is a series of numbers where the next number is found by adding up the two numbers before it. It starts from 0 and 1 and continues indefinitely. Each number in the sequence is called a Fibonacci number. This sequence has extensive applications in computer science, mathematics, and even in natural phenomena.
In this article, you will learn how to generate and display the Fibonacci sequence in Python using several methods. Explore straightforward loops, recursive functions, and advanced Python features to efficiently produce the sequence for different needs.
Generating Fibonacci Sequence Using a Loop
Simple Iterative Approach
Define the number of terms you need in the sequence.
Use a simple
for
loop to generate each term sequentially.pythondef fibonacci(num_terms): a, b = 0, 1 for _ in range(num_terms): yield a a, b = b, a + b # Print the first 10 Fibonacci numbers for value in fibonacci(10): print(value)
This function
fibonacci
uses tuple unpacking to update the values ofa
andb
. Theyield
keyword makesfibonacci
a generator, outputting one Fibonacci number per loop iteration.
Efficiency Considerations
- Recognize that looping is efficient for generating Fibonacci numbers up to a moderate sequence length.
- Consider system memory and execution time for very large sequences.
Recursive Method to Generate Fibonacci Numbers
Basic Recursive Function
Write a function that calls itself to calculate each number in the series.
Manage the base cases for the first two numbers of the sequence.
pythondef fibonacci_recursive(n): if n <= 0: return 0 elif n == 1: return 1 else: return fibonacci_recursive(n-1) + fibonacci_recursive(n-2) # Example to print the 10th Fibonacci number print(fibonacci_recursive(10))
This recursive function calculates the Fibonacci number by breaking down each number into the sum of the two preceding numbers, except for the first two numbers which are predefined.
Limitations of Recursion
- Understand that the recursive method has a significant performance drawback due to repeated calculations.
- Recognize potential for stack overflow with large numbers because of deep recursion.
Using Python's Advanced Features
Using List Comprehensions
Utilize Python’s list comprehension to create a list of Fibonacci numbers in a compact form.
Implement mathematical induction in one line of code.
pythonnum_terms = 10 fib_series = [0, 1] + [sum([fib_series[-2], fib_series[-1]]) for _ in range(2, num_terms)] print(fib_series)
This one-liner expands the Fibonacci series list by summing the last two elements of the list repetitively until the specified number of terms is reached.
Using Memoization with Decorators
Apply the memoization technique to optimize the recursive Fibonacci function.
Use Python's
functools.lru_cache
for caching previous results.pythonfrom functools import lru_cache @lru_cache(maxsize=None) def fibonacci_memo(n): if n < 2: return n else: return fibonacci_memo(n-1) + fibonacci_memo(n-2) # Example to print the 10th Fibonacci number using memoization print(fibonacci_memo(10))
The
lru_cache
decorator stores results of expensive recursive calls and reuses them when the same inputs occur again, significantly speeding up the computation.
Conclusion
Producing the Fibonacci sequence in Python can be approached in several ways, each beneficial for different scenarios. Loop methods provide straightforward solutions suitable for small to medium tasks. Recursive solutions, while elegant, may require optimizations like memoization to be practical for larger sequences. Python's powerful syntax and standard libraries support various methodologies to handle complex calculations efficiently. Implement these approaches to enhance your understanding of sequence generation, and select the method that best serves your specific programming needs.
No comments yet.