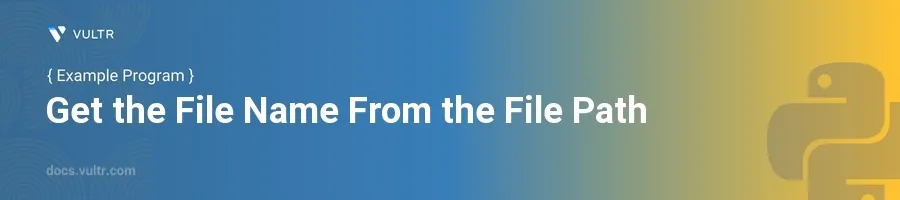
Introduction
Extracting the filename from a given file path is a common task in Python programming, especially when handling file I/O operations. Whether you are working with data processing scripts, managing logs, or organizing files, knowing how to get the file name from a path in Python is crucial for efficient manipulation and processing.
In this article, you will learn how to extract the file name from a file path in Python using practical examples. You'll explore methods using built-in libraries such as os
and pathlib
, which ensure your code remains portable and clean across different operating systems.
Using the os Module
Get File Name with os.path.basename
Import the
os
module, which provides a portable way of using operating system dependent functionality.Use the
os.path.basename()
function to extract the filename from a full path.pythonimport os file_path = '/home/user/data/example.txt' file_name = os.path.basename(file_path) print(file_name)
This code snippet imports the
os
module and usesos.path.basename()
to getexample.txt
from the givenfile_path
. Theos.path.basename()
function parses the path and returns only the final component, which is typically the file name.
Handle Edge Cases
Consider file paths that end with a slash, which might represent a directory rather than a file.
Use
os.path.basename()
in conjunction with other functions if necessary to ensure correct file name extraction.pythondirectory_path = '/home/user/data/' # Attempt to extract filename file_name = os.path.basename(directory_path) print("Extracted Name:", file_name)
Since
directory_path
here ends with a slash,os.path.basename()
returns an empty string, highlighting the importance of validating paths when extracting filenames in Python.
Using the pathlib Module
Extract Filename with pathlib
Import the
pathlib
module, which provides object-oriented file path handling.Use
Path.name
from thepathlib
module to get the file name.pythonfrom pathlib import Path file_path = Path('/home/user/data/example.txt') file_name = file_path.name print(file_name)
In this example, creating a
Path
object from the file path allows access to various properties and methods, where.name
directly provides the file name. This approach is modern and Pythonic for handling file paths.
Handling Paths Uniformly
Use
pathlib
to manipulate file paths in an OS-independent manner.Use
stem
for the filename without extension andsuffix
for the extension.pythonfile_path = Path('/home/user/data/example.txt') file_stem = file_path.stem file_extension = file_path.suffix print("File name without extension:", file_stem) print("File extension:", file_extension)
This snippet demonstrates how to extract the filename in Python from its extension using
stem
andsuffix
, enhancing the handling of file attributes separately. This method is useful for many applications, such as file sorting and filtering based on file type.
Conclusion
Extracting a filename from a file path in Python is essential for file operations, system administration, and data processing tasks. The os
module provides os.path.basename()
, a straightforward method for filename extraction, while the pathlib
module offers a more modern, object-oriented way to handle file paths in Python. By mastering these methods, you can efficiently manage file-related operations across different platforms and applications.
No comments yet.