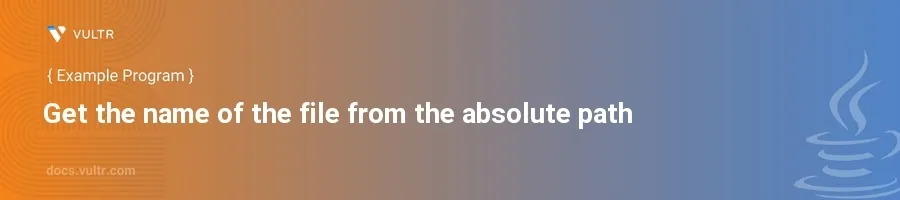
Introduction
When working with file systems in Java, it's often necessary to extract specific portions of a file's path. For instance, getting just the name of a file from its absolute path is a common task in Java, particularly useful in applications involving file manipulation, logging, or displaying file information in user interfaces.
In this article, you will learn how to get the file name from a path in Java using multiple approaches. We will explore methods using the File
class from java.io
and the Path
class from java.nio.file
. These techniques will help you extract a file name, get the full file path, and work with file directories efficiently.
Extracting File Name in Java Using the File Class
The File
class in Java provides a straightforward way to obtain the file name from a given path. The getName()
method allows you to extract only the file name, excluding the directory path.
Retrieve the File Name from an Absolute Path
Create an instance of the
File
object, passing the absolute path as a string to the constructor.Call the
getName()
method on theFile
object to obtain just the file name.javaimport java.io.File; public class FileNameExtractor { public static void main(String[] args) { File file = new File("/path/to/your/filename.txt"); String fileName = file.getName(); System.out.println("File name: " + fileName); } }
This code snippet creates a
File
object for the specified absolute file path in Java and then retrieves the name of the file usinggetName()
. The output will befilename.txt
, which is the file name.
Using the Path Class and the Files Class
With Java 7 and newer, the java.nio.file
package (New Input/Output 2) introduces a more comprehensive API for file handling. The Path
class, combined with the Files
class, provides a powerful way to deal with more complex file paths in Java.
Utilizing the Path Class to Get the File Name
Use
Paths.get()
to convert the string representation of the path into aPath
object.Obtain the name of the file by calling
getFileName()
method on thePath
object.javaimport java.nio.file.Path; import java.nio.file.Paths; public class FileNameExtractorNIO { public static void main(String[] args) { Path path = Paths.get("/path/to/your/filename.txt"); Path fileName = path.getFileName(); System.out.println("File name: " + fileName); } }
Here, the
Paths.get()
method parses the absolute path and converts it into aPath
object. ThegetFileName()
method then extracts the file name from thePath
object. The result is aPath
object, not a string, but its textual representation will display the file name:filename.txt
.
Advanced Usage: Filtering and Processing Paths
In real-world applications, extracting a file name might be part of a larger file processing or filtering task. You might need to get all file names in a directory or filter files by their extension. You can use streams with the Files API to handle complex scenarios like this efficiently.
Example: Filtering Specific File Types
Suppose you need to list all .txt
files in a directory and extract their names only.
Stream the directory contents using
Files.list()
.Filter the files by their extension.
Extract and print each file name.
javaimport java.io.IOException; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; import java.util.stream.Stream; public class FileFilterExample { public static void main(String[] args) { Path dirPath = Paths.get("/path/to/your/directory"); try (Stream<Path> paths = Files.list(dirPath)) { paths.filter(path -> path.toString().endsWith(".txt")) .map(Path::getFileName) .forEach(System.out::println); } catch (IOException e) { e.printStackTrace(); } } }
This code lists all
.txt
files in the specified directory and extracts just their names. The result is output directly to the console, enabling quick visualization of all text files in the directory.
Conclusion
Extracting the name of a file from its absolute file path in Java can be done using either the File
or Path
classes. Whether you're managing file paths using Java's traditional IO
library or leveraging the newer NIO
classes, both approaches provide robust methods for handling file paths efficiently.
If you need to get the file name from a path in Java, retrieve the absolute path in Java, or work with directories, these methods will serve you well. Mastering these file-handling techniques will enhance your Java applications and make them more dynamic in processing file operations effectively.
No comments yet.