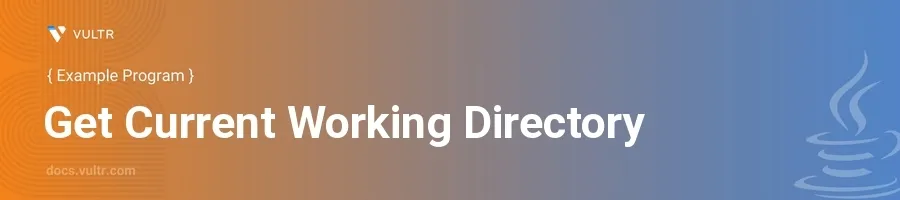
Introduction
When working with Java, obtaining the current working directory can be crucial, especially when handling file operations without absolute paths. This approach ensures that your program behaves predictably across different environments and systems.
In this article, you will learn how to retrieve the current working directory in Java. You'll explore methods involving system properties and the newer Path
API from Java NIO (New Input/Output), which was introduced to complement the old File I/O operations.
Retrieving Current Working Directory in Java
Using System Properties
System properties provide an easy way to access system-wide information about the current environment, including the user's directory. Java uses these properties to interact seamlessly with the host environment.
Access the system property that stores the current working directory.
javaString workingDir = System.getProperty("user.dir"); System.out.println("Current working directory: " + workingDir);
This snippet retrieves the current working directory using the key
"user.dir"
, which Java sets during the application launch. The program prints the directory to the console, providing a straightforward result showing where your Java application executes from.
Utilizing Java NIO
Java NIO provides a more modern and flexible approach to dealing with I/O operations in Java. The Path
class, part of Java NIO, is robust for path operations.
Use the
Paths
class from Java NIO to get the current working directory.javaPath currentPath = Paths.get("").toAbsolutePath(); System.out.println("Current working directory using Path: " + currentPath);
Here,
Paths.get("")
obtains a Path object representing the current directory, andtoAbsolutePath()
resolves it into an absolute path. This method ensures that you get a complete path, regardless of the context in which your Java program is executed.
Conclusion
Retrieving the current working directory in Java is essential for applications that depend on relative file paths. Whether you choose to utilize System properties or Java NIO's Path
class, both methods offer reliable ways to achieve this, with Path
providing a more modern and comprehensive solution. Apply these techniques in your Java applications to handle file directories more effectively, ensuring your program remains portable and environment-independent.
No comments yet.