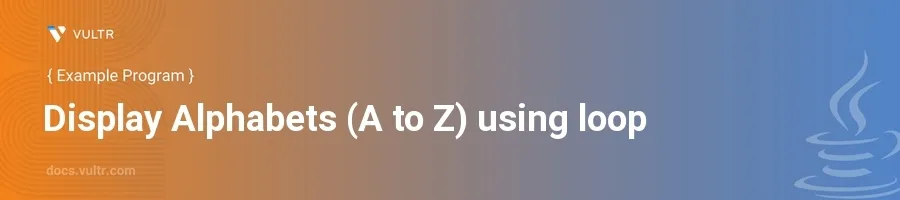
Introduction
The need to display a sequence of characters, such as alphabets from A to Z, arises in various programming scenarios, particularly in educational purposes, data processing, and user interface development. Java, being a robust programming language, provides several ways to achieve this using loops.
In this article, you will learn how to create a Java program that displays alphabets from A to Z using different types of loops. Each example will demonstrate a practical use-case of loops like for
and while
to iterate over character values.
Displaying Alphabets Using a For Loop
Using the for
Loop
Understand that a
for
loop allows precise control over the iteration process.Initialize a character variable in the loop and use its ASCII values to control the loop execution.
Increment the character in each iteration.
javapublic class AlphabetDisplay { public static void main(String[] args) { for (char ch = 'A'; ch <= 'Z'; ch++) { System.out.print(ch + " "); } } }
This code initializes
ch
as 'A' and increments it in each iteration until it reaches 'Z'. Each character is printed on the same line separated by a space.
Using the ASCII Values
Emphasize that ASCII values can be directly manipulated in Java.
Start with the ASCII value of 'A' and loop until 'Z'.
Convert each ASCII value back to a character for display.
javapublic class AlphabetDisplayASCII { public static void main(String[] args) { for (int i = 65; i <= 90; i++) { System.out.print((char)i + " "); } } }
Here,
i
iterates from 65 (ASCII value of 'A') to 90 (ASCII value of 'Z'). The cast(char)i
converts the integeri
to its corresponding ASCII character.
Displaying Alphabets Using a While Loop
Using the while
Loop
Use a
while
loop for a simpler, condition-based iteration without an explicit iteration variable.Initialize a character outside the loop and increment it after each print statement within the loop.
javapublic class AlphabetDisplayWhile { public static void main(String[] args) { char ch = 'A'; while (ch <= 'Z') { System.out.print(ch + " "); ch++; } } }
In this snippet,
ch
starts at 'A' and is incremented in each iteration of the loop. Thewhile
loop continues as long asch
is less than or equal to 'Z'.
Conclusion
Displaying the alphabets from A to Z in Java can be achieved using different loop constructs such as for
and while
. The examples provided demonstrate how to leverage loop mechanics effectively to iterate over a range of characters seamlessly. These basic constructs form the foundation for more complex logic in Java programming, including data validation, text processing, and more. By mastering these loop mechanisms, you enhance your ability to manipulate and control the flow of execution in Java applications.
No comments yet.