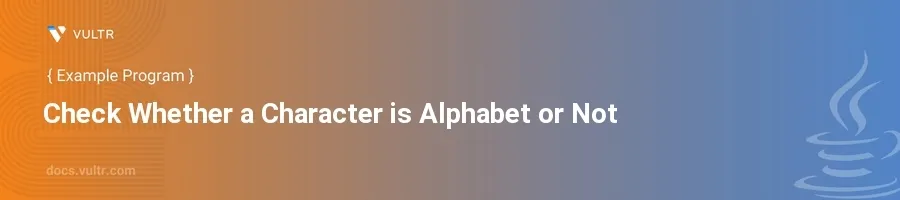
Introduction
Java offers multiple ways to determine if a specific character is part of the alphabet. This ability is crucial for input validation, parsing, and various text-processing applications where character identification plays a key role. Whether you are building a user input form or analyzing text data, identifying alphabetic characters efficiently can streamline your operations and enhance performance.
In this article, you will learn how to check if a character is part of the alphabet in Java. Explore different methods, including using character checks and regular expressions, to implement this functionality effectively in your Java programs.
Utilizing Character Methods
Using Character.isLetter()
Import the
Character
class.Utilize the
Character.isLetter(char ch)
method to determine if the character is an alphabetic letter. This method returnstrue
if the character is a letter.javapublic class AlphabetCheck { public static void main(String[] args) { char ch = 'a'; boolean isAlphabet = Character.isLetter(ch); System.out.println(ch + " is an alphabet: " + isAlphabet); } }
This code checks if the character
'a'
is an alphabet. Since'a'
is a letter,Character.isLetter(ch)
returnstrue
.
Using Character.isAlphabetic()
Understand that
Character.isAlphabetic(int codePoint)
is another method that checks if the specified character (Unicode code point) is alphabetic.Implement this method, which also considers letters from non-Latin scripts.
javapublic class AlphabetCheck { public static void main(String[] args) { char ch = 'ñ'; boolean isAlphabet = Character.isAlphabetic(ch); System.out.println(ch + " is an alphabet: " + isAlphabet); } }
Here, the character
'ñ'
is checked. TheCharacter.isAlphabetic(ch)
method returnstrue
because'ñ'
is considered an alphabetic character in many extended Latin alphabets.
Using Regular Expressions
Simple Regex Check
Use a regular expression to determine if a character is an alphabet letter.
Apply the regular expression using the
String.matches(String regex)
method.javapublic class AlphabetCheck { public static void main(String[] args) { char ch = 'A'; boolean isAlphabet = ("" + ch).matches("[a-zA-Z]"); System.out.println(ch + " is an alphabet: " + isAlphabet); } }
This example converts the character to a string and checks if it matches the regex
[a-zA-Z]
, which includes any uppercase or lowercase letter from A to Z. Since'A'
matches, it confirms that it is an alphabetic character.
Conclusion
Checking if a character is part of the alphabet in Java can be accomplished using simple character checks or regular expressions. The Character
class offers methods like Character.isLetter()
and Character.isAlphabetic()
that handle most use cases, including extended alphabetic sets. For stricter or customized checks, regular expressions provide a powerful alternative. By choosing the right method based on the specific requirements and context of your program, you effectively handle character validation tasks in Java applications.
No comments yet.