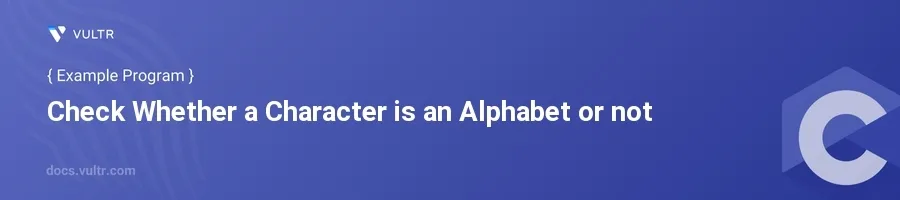
Introduction
Determining whether a given character is an alphabet character is a fundamental exercise in programming that introduces conditional statements and character handling in C. This kind of functionality is crucial in applications that process textual data, where determining the type of character can influence the flow of data handling or user input validation.
In this article, you will learn how to implement a program in C that checks if a particular character is an alphabet letter. Explore practical examples that demonstrate the use of conditional operators and ASCII values to achieve this task effectively.
Implementing the Alphabet Check in C
Use of Conditional Statements
Understand the use of ASCII values for characters:
- Characters 'A' to 'Z' have ASCII values from 65 to 90.
- Characters 'a' to 'z' have ASCII values from 97 to 122.
Write a C program using if-else statements to check for alphabets:
c#include <stdio.h> int main() { char c; printf("Enter a character: "); scanf("%c", &c); if ((c >= 'A' && c <= 'Z') || (c >= 'a' && c <= 'z')) printf("%c is an alphabet.\n", c); else printf("%c is not an alphabet.\n", c); return 0; }
In this program, the user inputs a character, and the
if
statement checks whether the ASCII value of the character falls within the ranges of uppercase or lowercase alphabetic characters. If it does, it confirms that the character is an alphabet. Otherwise, it is not.
Enhancing with Ternary Operator
Learn how a ternary operator can simplify the code:
c#include <stdio.h> int main() { char c; printf("Enter a character: "); scanf("%c", &c); char *result = (c >= 'A' && c <= 'Z') || (c >= 'a' && c <= 'z') ? "is an alphabet." : "is not an alphabet."; printf("%c %s\n", c, result); return 0; }
This version of the program does the same check but uses a ternary operator to assign the output message to a pointer variable,
result
. This reduces the lines of code and makes the conditional logic more concise.
Conclusion
By using simple conditional statements or the ternary operator in C, you can effectively determine whether a character is an alphabetical letter. The approaches shown not only help in validating input but also serve as a good practice for understanding basic concepts of character data types and ASCII values. Apply these techniques in your text-processing applications to enhance data validation and user interactions. Utilizing the ASCII table for such checks ensures that your logic remains clear and efficient.
No comments yet.