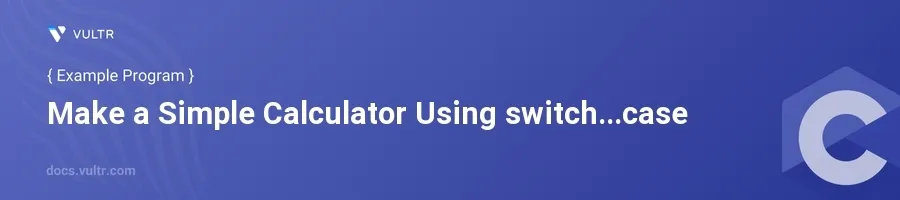
Introduction
A simple calculator is one of the fundamental projects a beginner might handle while learning programming. It encapsulates the basic operations such as addition, subtraction, multiplication, and division. Using switch...case
in C programming, you can efficiently handle multiple operations by leveraging conditional checks.
In this article, you will learn how to develop a simple calculator in C using the switch...case
statement. You'll see how to set up the basic structure of a program to handle different mathematical operations based on user input, without the complexity of lengthy if-else conditions.
Building a Simple Calculator
Initialize and Capture User Inputs
Begin by including the necessary header files and declaring the main function.
Initialize variables to store operand values and the operator.
Prompt the user to input two operand values and the operator.
c#include<stdio.h> int main() { double num1, num2; char operator; printf("Enter first number: "); scanf("%lf", &num1); printf("Enter second number: "); scanf("%lf", &num2); printf("Enter the operator (+, -, *, /): "); scanf(" %c", &operator);
This section of code sets up the necessary variables and captures inputs from the user. It’s important to include a space before
%c
in thescanf
function to skip over any whitespace characters left in the input buffer.
Process the Operation Using switch...case
Insert a
switch
block to handle operations based on the operator input.Define cases for each operator: '+', '-', '*', and '/'. Remember to handle division by zero as a special case.
Print the result of the operation.
cswitch(operator) { case '+': printf("%.2lf + %.2lf = %.2lf\n", num1, num2, num1 + num2); break; case '-': printf("%.2lf - %.2lf = %.2lf\n", num1, num2, num1 - num2); break; case '*': printf("%.2lf * %.2lf = %.2lf\n", num1, num2, num1 * num2); break; case '/': if(num2 != 0.0) printf("%.2lf / %.2lf = %.2lf\n", num1, num2, num1 / num2); else printf("Division by zero error!\n"); break; default: printf("Invalid operator!\n"); } return 0; }
In this segment, the
switch
statement directs the execution flow to different blocks based on the operator. Each case corresponds to an arithmetic operation, handling the computation and output in one step. The code also addresses error handling for division by zero and an invalid operator input.
Conclusion
Using the switch...case
statement in C to build a simple calculator allows for clear, efficient, and manageable coding of operations based on user input. This approach is cleaner and more scalable than multiple if-else statements, especially as the complexity of the conditions increases. By implementing the steps demonstrated, you've developed a robust program capable of basic arithmetic operations. This foundational knowledge can be extended to create more complex applications, enhancing both your problem-solving and programming skills.
No comments yet.