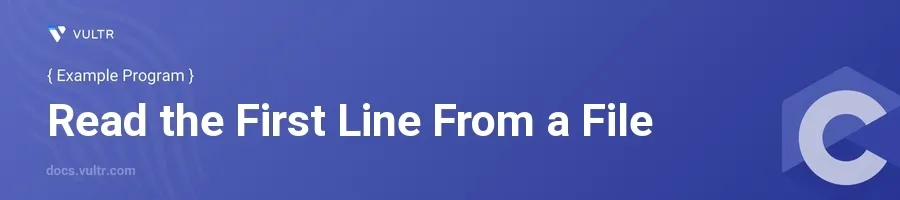
Introduction
Reading from a file is a foundational task in C programming that enables applications to ingest and process data stored on disk. This functionality is particularly important for applications that require initial configuration or data loading before execution. Handling files in C is straightforward, thanks to its standard library functions that provide robust tools for file operations.
In this article, you will learn how to create a simple C program that reads the first line from a file. You will explore different examples that demonstrate how to open a file, read its first line, and handle any potential errors during the process.
Opening and Reading a File in C
Setup a File Pointer and Open the File
Include the necessary headers.
Declare a file pointer.
Use
fopen()
to open the file in read mode.c#include <stdio.h> #include <stdlib.h> int main() { FILE *filePointer; filePointer = fopen("example.txt", "r"); if (filePointer == NULL) { perror("Error opening file"); return EXIT_FAILURE; } }
This snippet initializes a file pointer and attempts to open "example.txt" in read mode. If the file cannot be opened, it prints an error message and exits the program.
Reading the First Line from the File
Declare a buffer to store the line read from the file.
Use
fgets()
to read the first line.cchar buffer[1000]; // Adjust size as needed if (fgets(buffer, 1000, filePointer) != NULL) { printf("First line: %s", buffer); } else { perror("Error reading from file"); }
Here,
fgets()
is used to read up to 999 characters or until a newline or EOF is reached. This function places the first line from the file into thebuffer
, and it prints the line to the console.
Close the File
Release the file resource.
Add error handling if the file does not close properly.
cif (fclose(filePointer) != 0) { perror("Error closing file"); return EXIT_FAILURE; } return EXIT_SUCCESS;
This code attempts to close the file using
fclose()
. It checks for errors during the closure process, printing an error message if necessary.
Conclusion
Reading the first line from a file in C is a straightforward task that requires setting up a file pointer, using functions from the standard library to handle file operations, and ensuring proper error handling. The functionalities provided by fopen()
, fgets()
, and fclose()
offer a simple yet powerful way to work with file data in C programs. As illustrated in the examples, managing file operations meticulously is crucial for stable and reliable application performance. With this knowledge, effectively manage file inputs in your C programs to enhance their interactivity and functionality.
No comments yet.