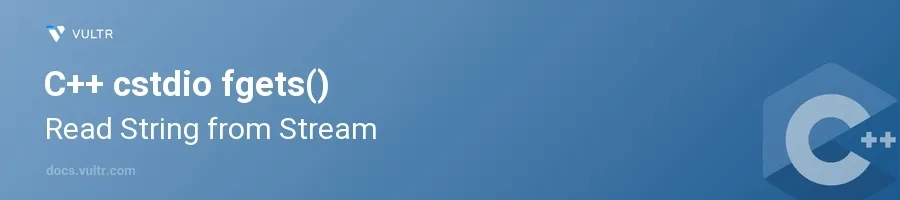
Introduction
The fgets()
function in C++ is a robust tool that reads a line of text, or a specified amount of characters, from a stream and stores it into the string buffer you pass as an argument. It's part of the <cstdio>
library, which is generally used for input and output operations in C-style programming. Though primarily a C function, fgets()
is often utilized in C++ programming for handling legacy code or for situations where simplistic buffer-based input is suitable.
In this article, you will learn how to effectively utilize the fgets()
function to read data from streams in C++. Explore how to handle different types of input sources such as files or standard input (stdin), and how to manage potential pitfalls like buffer overflows and null characters.
Reading Strings from Standard Input
Basic Usage of fgets()
Declare a buffer of characters where the input will be stored.
Use
fgets()
to read a line fromstdin
.cpp#include <cstdio> char buffer[100]; if (fgets(buffer, 100, stdin)) { printf("You entered: %s", buffer); } else { printf("Error reading input."); }
This code reads up to 99 characters from the standard input. The
fgets()
function ensures the string is null-terminated and will stop reading if it encounters a newline character, storing it in the buffer. If an error occurs or end-of-file is reached without any characters being read, thefgets()
returns a null pointer.
Ensuring Buffer Safety
Specify the correct limit for the buffer size to prevent overflow.
Always check the return value of
fgets()
to handle errors and end-of-file scenarios gracefully.In the example above, the buffer size is limited to 100 characters, which protects from overflow as
fgets()
includes the null-terminating character automatically.
Reading Strings from a File
Using fgets() with File Streams
Open the desired file using
fopen()
.Use
fgets()
similarly as reading fromstdin
.cpp#include <cstdio> FILE *file = fopen("example.txt", "r"); if (file) { char buffer[256]; while (fgets(buffer, 256, file)) { printf("%s", buffer); } fclose(file); } else { printf("Failed to open file."); }
Here,
fgets()
reads each line from the file referenced by theFILE*
pointer until the end-of-file is reached. The buffer size of 256 ensures that longer lines are handled correctly up to 255 characters.
Conclusion
The fgets()
function offers a reliable way to read strings from streams in C++, particularly in scenarios demanding compatibility with C-style input/output functions. By understanding its behavior and limitations, such as the inclusion of newline characters in the output and its handling of buffer limits, you can safely implement fgets()
in various input-reading situations. Utilize this function to enhance the robustness of file operations and user input handling in your applications, ensuring all operations respect buffer boundaries and gracefully handle potential errors or end-of-stream conditions.
No comments yet.