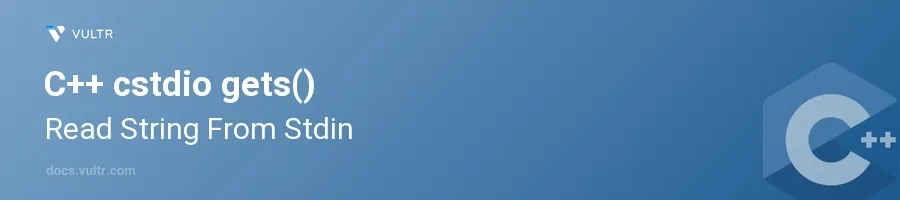
Introduction
The gets()
function in C++ is a standard library function housed within the <cstdio>
header, primarily used for reading strings from standard input (stdin). It captures an entire line from stdin, up to but not including the newline character, and stores it into a buffer provided by the user. Despite its ability to read input from stdin in C++, gets()
has been deprecated due to safety concerns, primarily buffer overflow vulnerabilities.
In this article, you will learn how to use the gets()
function to read input strings from the user in C++. Explore how gets()
operates, its associated risks, and alternatives that can be used to achieve similar functionality safely.
Understanding gets()
in C++ for Reading Strings from Stdin
Basic Usage of gets()
Include the
<cstdio>
header in your C++ program.Declare a buffer to store the input string from stdin.
Use the
gets()
function to read the input from stdin.cpp#include <cstdio> int main() { char str[100]; printf("Enter a string: "); gets(str); printf("You entered: %s\n", str); return 0; }
This code snippet sets up a buffer
str
capable of holding up to 99 characters (allowing space for the null terminator). Thegets()
function reads a line of text from the user and stores it in this buffer.
The Risks of Using gets()
in C++ for Reading Input from Stdin
Realize that
gets()
does not perform bounds checking.Recognize the risk of buffer overflow in C++, which can lead to security vulnerabilities.
When using
gets()
, if the input exceeds the buffer size, it can overwrite adjacent memory areas, causing program crashes or security exploits. That’s why it’s discouraged in modern C++ programs to read input from stdin.
Safe Alternatives to gets()
to Read Strings from Stdin in C++
Using fgets()
for Safer Input
Use
fgets()
to read strings from stdin safely in C/C++ with buffer size limitation.Specify the buffer, the maximum size, and the stdin stream as arguments to
fgets()
.cpp#include <cstdio> int main() { char str[100]; printf("Enter a string: "); fgets(str, sizeof(str), stdin); printf("You entered: %s", str); // `fgets()` includes the newline character in the buffer return 0; }
Here,
fgets()
reads up to 99 characters and includes safety for preventing buffer overflow. Note thatfgets()
keeps the newline character if it reads it before reaching the buffer limit.
Using C++ Strings for Dynamic Handling
Utilize C++
std::string
andstd::getline()
for dynamic input handling.Include the
<iostream>
and<string>
headers for this purpose.cpp#include <iostream> #include <string> int main() { std::string input; std::cout << "Enter a string: "; std::getline(std::cin, input); std::cout << "You entered: " << input << std::endl; return 0; }
This approach uses C++ standard library features, providing more flexibility and safety compared to
gets()
or evenfgets()
. It’s especially useful when reading strings, lines, or matrices from stdin in C++.
Conclusion
While the gets()
function in C++ offers a straightforward method to read strings from stdin, its usage is strongly discouraged due to the inherent risks of buffer overflow. Explore and utilize safer alternatives like fgets()
and C++ strings with std::getline()
for robust and secure input handling in your applications. By adhering to these safer practices, you ensure that your applications remain robust, secure, and maintainable.
No comments yet.