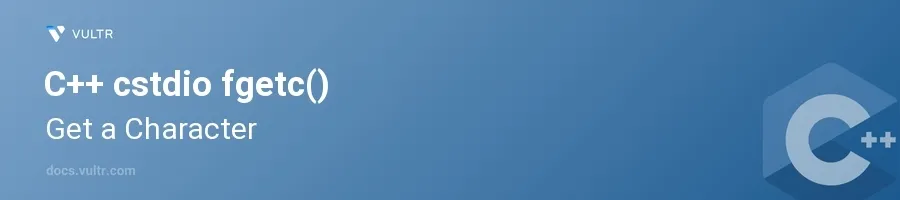
Introduction
The fgetc()
function in C++ is a standard library function used for reading a single character from a file. This function is part of the C standard input and output library <cstdio>
, and it is extremely useful when you need to read characters one at a time from a file, allowing for detailed processing of file contents.
In this article, you will learn how to effectively use the fgetc()
function to read characters from files in C++. Discover how to implement this function in various scenarios, including handling end-of-file conditions and error detection.
Understanding fgetc()
Basic Usage of fgetc()
Include the
<cstdio>
library in your C++ program.Open a file using
fopen()
.Use
fgetc()
to read characters.Handle the end-of-file (EOF) and possible errors.
Close the file using
fclose()
.cpp#include <cstdio> int main() { FILE *file = fopen("example.txt", "r"); if (file == NULL) { perror("Failed to open file"); return 1; } int ch; while ((ch = fgetc(file)) != EOF) { putchar(ch); } fclose(file); return 0; }
The code opens a file named "example.txt" and reads it character by character. If
fgetc()
reaches the end of the file, it returnsEOF
. Each character read is output to the console until EOF is reached. Finally, the file is closed.
Error Handling with fgetc()
Be aware that
fgetc()
returnsEOF
not only at the end of the file but also if a read error occurs.cppif (feof(file)) { printf("End of file reached.\n"); } if (ferror(file)) { printf("Error reading from file.\n"); }
After completion of the reading process or as part of error-checking routines, check if the EOF is due to reaching the end of the file or due to an error. This ensures that the program can differentiate between a normal completion and an error scenario.
Advanced Techniques
Using fgetc() inside Conditional Statements
Use
fgetc()
to perform operations based on the characters read.cppchar c; while ((c = fgetc(file)) != EOF) { if (c == '\n') { // Process new line } else { // Process other characters } }
This snippet shows how you might use
fgetc()
in a more complex logic flow, processing different characters differently, for example, handling new lines or specific delimiters.
Building a Function Using fgetc()
Create a custom function to encapsulate functionality using
fgetc()
.cppvoid printFileContent(FILE *file) { int ch; while ((ch = fgetc(file)) != EOF) { putchar(ch); } }
Encapsulating
fgetc()
usage within a function streamlines code, especially when similar file reading operations are needed multiple times within your application.
Conclusion
The fgetc()
function in C++ serves as a vital tool for file reading operations, where control over character-by-character processing is needed. Leveraging fgetc()
effectively ensures that your programs can handle files robustly, accommodating precise control over input handling, error checking, and data processing. By mastering the usage of fgetc()
, you solidify your understanding of file I/O in C++ and enhance your capability to tackle more complex programming challenges involving file manipulation.
No comments yet.