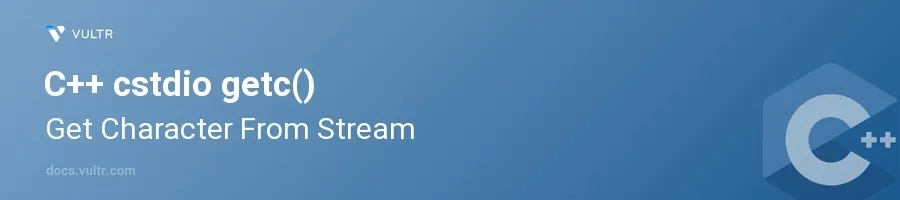
Introduction
The getc()
function in C++ is a standard library function employed in the C stdio library for reading a single character from a file or a stream. This function plays a crucial role in file handling, where it allows developers to read characters sequentially from a stream, making it possible to process text files or input data character by character.
In this article, you will learn how to use the getc()
function effectively in various scenarios. Discover strategies to handle files correctly, manage error states in your code, and understand how getc()
interacts with different types of streams, such as those connected to files or standard input.
Getting Started with getc()
Reading a Single Character from stdin
Include the necessary headers and use
getc()
to read a character from the standard input.cpp#include <cstdio> int main() { printf("Enter a character: "); int c = getc(stdin); printf("You entered: %c\n", c); return 0; }
This code snippet reads a single character from the standard input (usually the keyboard) and prints it. The
getc()
function returns the character as an integer, which can be typecast to a char directly.
File I/O with getc()
Ensure the correct header file is included and open a file for reading.
Use
getc()
to read characters from the file until the end of the file is reached.cpp#include <cstdio> int main() { FILE *file = fopen("example.txt", "r"); if (file == NULL) { perror("Failed to open file"); return 1; } int c; while ((c = getc(file)) != EOF) { putchar(c); } fclose(file); return 0; }
In the example above, the program opens a file named
"example.txt"
and reads from it character by character usinggetc()
. It prints each character to the standard output until it reaches the End of File (EOF). After reading, it closes the file to free up resources.
Handling Errors with getc()
Recognize that
getc()
returns EOF not only at the end of a file but also on an error.Check for errors during file read operations appropriately.
cpp#include <cstdio> int main() { FILE *file = fopen("example.txt", "r"); if (file == NULL) { perror("Failed to open file"); return 1; } int c; while (true) { c = getc(file); if (c == EOF) { if (feof(file)) { printf("End of file reached.\n"); } if (ferror(file)) { printf("Error reading from file.\n"); break; } } else { putchar(c); } } fclose(file); return 0; }
This example adds error checking to the file reading loop. It differentiates between reaching the end of the file and an error occurring during file reading. This distinction helps to avoid misunderstandings when EOF is returned and is critical for robust error handling in applications where file integrity is important.
Conclusion
The getc()
function is a simple yet powerful tool in C++ for stream-based character input. It is essential for reading data sequentially from files or standard input. By integrating getc()
effectively into your programs, you improve how inputs are handled, making your applications capable of processing data accurately and efficiently. Implement these functionalities in your projects to read and manage file and input data effectively.
No comments yet.