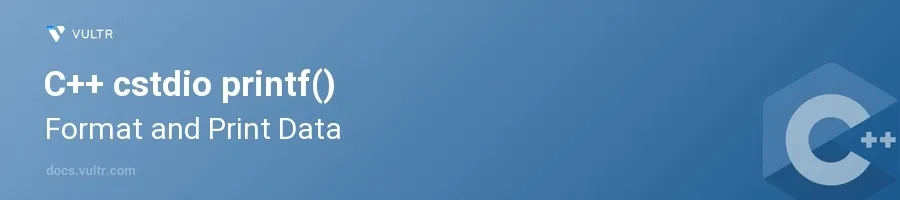
Introduction
The printf()
function in C++ originates from the C standard input and output library, commonly referred to as <cstdio>
. This function enables formatted output to the console, allowing developers to control the display of variables and text with specific formats like integers, floating points, characters, and strings. Its flexibility and control over output formatting make it a staple in both C and C++ programming for developing user-friendly console applications.
In this article, you will learn how to employ the printf()
function in C++ for various data formatting needs. Explore how to manage different data types and use format specifiers effectively to produce clear and organized output. Additionally, discover some common pitfalls and how to avoid them, ensuring your console output is both accurate and informative.
Basics of Using printf()
Basic Syntax and Format Specifiers
Include the
cstdio
library at the beginning of your code.cpp#include <cstdio>
Utilize
printf()
by passing a string with format specifiers that correspond to the supplied variables.cppint main() { printf("Hello, World!\n"); return 0; }
This code simply prints "Hello, World!" followed by a new line. The
\n
is a newline character that moves the cursor to the next line.
Formatting Integer Types
Use
%d
or%i
for standard decimal integers.cppint age = 25; printf("Age: %d years old\n", age);
This snippet will output:
Age: 25 years old
. Here,%d
is replaced by the value of the integerage
.Handle unsigned integers with
%u
.cppunsigned int items = 300; printf("Items in stock: %u items\n", items);
The
%u
specifier is used for unsigned integers, displayingItems in stock: 300 items
in the console.
Formatting Floating Point Numbers
Use
%f
for floating point numbers, and%e
or%E
for exponential format.%g
or%G
automatically chooses between fixed-point or exponential.cppdouble balance = 3200.75; printf("Account Balance: $%.2f\n", balance);
%f
formats the floating point numberbalance
to two decimal places, resulting inAccount Balance: $3200.75
.
Printing Strings
Use
%s
for strings.cppchar name[] = "John Doe"; printf("Name: %s\n", name);
The
%s
specifier inserts the stringname
into the output, which producesName: John Doe
.
Combining Multiple Data Types
You can mix different format specifiers in a single
printf()
statement to output varied data types.cppchar dept[] = "Engineering"; int empCount = 150; printf("Department: %s - Employees: %d\n", dept, empCount);
This outputs
Department: Engineering - Employees: 150
, using%s
for string and%d
for integer.
Common Mistakes and Troubleshooting
- Ensure the format specifier matches the type of the variable.
- Misusing a specifier can lead to unpredictable output or runtime errors, particularly if the memory layout or type expectations are not met.
- Pay close attention to the use of
unsigned
types versussigned
types.
Conclusion
The printf()
function from the C++ <cstdio>
library is invaluable for formatted console output. Mastering its format specifiers allows precise control over how data is presented, enhancing both the functionality and aesthetic of console-based applications. Always ensure the correct usage of format specifiers corresponding to the variable types. This practice prevents common errors and maintains the robustness and readability of your code. By incorporating these techniques, streamline the data presentation in your C++ applications efficiently.
No comments yet.