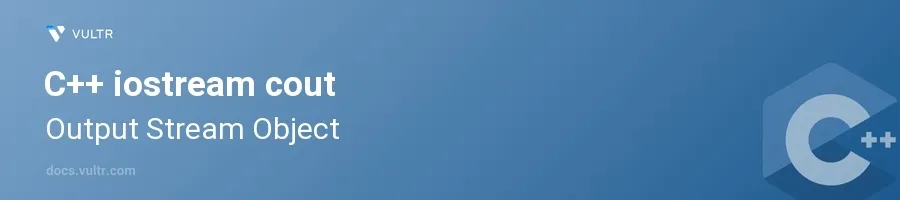
Introduction
The cout
object in C++ is part of the iostream library and stands as the primary mechanism for producing output to the standard output device, usually the screen. It's a tool used extensively for displaying text and variables to the console, making it an essential component for debugging and user interaction in C++ applications.
In this article, you will learn how to effectively utilize the cout
object to output various types of data. Understand how to manipulate output using cout
and explore advanced usages such as formatting output and integrating user-defined types.
Basic Usage of cout
Outputting Text and Numbers
Include the iostream library and utilize
cout
to output text and numbers.cpp#include <iostream> int main() { std::cout << "Hello, World!" << std::endl; std::cout << "The number is: " << 42 << std::endl; return 0; }
This code snippet demonstrates the basic usage of
cout
for printing strings and integers.std::endl
is used to add a new line after the output.
Combining Text and Variables
Declare and output variables using
cout
.cpp#include <iostream> int main() { int number = 15; std::cout << "Selected number is: " << number << std::endl; return 0; }
In this example, an integer variable
number
is declared, assigned the value15
, and then output usingcout
.
Advanced Output Formatting
Setting Width and Filling Characters
Use manipulators like
std::setw
andstd::setfill
to format the output.cpp#include <iostream> #include <iomanip> int main() { std::cout << std::setfill('*') << std::setw(10) << 77 << std::endl; return 0; }
With
std::setw(10)
,cout
will produce an output 10 characters wide, andstd::setfill('*')
fills the remaining characters with asterisks.
Controlling Precision for Floating-Point Numbers
Manipulate the precision of floating-point outputs.
cpp#include <iostream> #include <iomanip> int main() { double pi = 3.14159; std::cout << "Pi is approximately: " << std::setprecision(2) << pi << std::endl; return 0; }
This code sets the output precision of the floating-point number
pi
to 2 decimal places.
Conclusion
The cout
object from the C++ iostream library is a foundational tool for output in C++ programming. Whether you're outputting simple messages, or need precise control over formatting complex data types, cout
provides the functionality to meet these needs. Mastering cout
enhances the readability and usability of your C++ programs by allowing clear and formatted output on the console. Use the various techniques discussed to improve how you display data in your C++ applications, ensuring your output is both informative and visually appealing.
No comments yet.