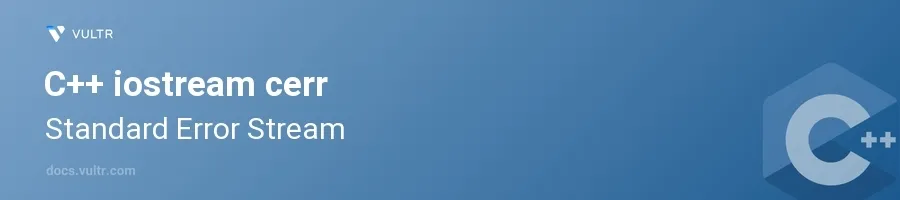
Introduction
cerr
in C++ is one of the standard iostream objects that is used to handle errors and output them to the standard error (stderr). Compared to cout
which outputs to the standard output (stdout), cerr
is unbuffered and ensures that error messages are displayed immediately, making it especially useful in debug and error handling scenarios where immediate feedback is crucial.
In this article, you will learn how to effectively use the cerr
stream in C++ programming. Explore when and why to use cerr
instead of cout
and how it integrates with standard practice in handling exceptions and error reporting in robust applications.
Understanding cerr for Error Output
Basic Usage of cerr
Include the iostream library.
Output a simple error message using
cerr
.cpp#include <iostream> int main() { cerr << "Error: Failed to open file." << endl; return 1; }
This snippet outputs an error message if a file fails to open. The message is sent to the standard error stream.
Comparing cerr with cout
Recognize that both
cerr
andcout
belong to the same iostream library, but they serve different purposes.Use
cerr
for errors to ensure immediate output.cpp#include <iostream> int main() { cout << "This is a regular message." << endl; cerr << "This is an error message." << endl; return 0; }
Here, the first message will be output to standard output, while the second, an error message, goes to standard error.
Error Handling with Exceptions
Understand how exception handling can be used together with
cerr
for robust error reporting.Encapsulate operations prone to failure in a try-catch block and use
cerr
in the catch section.cpp#include <iostream> #include <stdexcept> int main() { try { throw std::runtime_error("Intentional Error"); } catch (const std::exception& e) { cerr << "Caught exception: " << e.what() << endl; } return 0; }
This code throws an exception deliberately, and the corresponding catch block uses
cerr
to display the error message.
Best Practices Using cerr
- Utilize
cerr
exclusively for error messages and diagnostics that require immediate attention. - Keep the output to
cerr
minimal to ensure clarity and effectiveness of the error messages. - Combine
cerr
with exception handling for a comprehensive error recovery strategy that maintains reliable application behaviour.
Conclusion
The cerr
stream in C++ serves as a critical tool for error handling by ensuring that messages reach the user without delay, crucial for diagnosing issues during development or in production environments. Familiarize yourself with cerr
and integrate it into standard practices for handling errors in your C++ applications, complemented effectively by structured exception handling to provide clear, actionable feedback on issues as they occur. Through the appropriate application of techniques demonstrated, you enhance the robustness and user-friendliness of your software.
No comments yet.