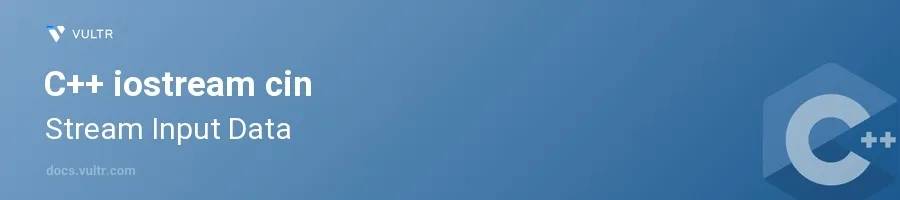
Introduction
The cin
object in C++ is a part of the iostream library and stands as one of the primary means for handling input in C++. Utilizing cin
, developers can capture data from the standard input device, commonly the keyboard, thereby allowing dynamic data input during program execution. This mechanism is crucial for interactive applications, such as those requiring user configuration or real-time calculations based on user entry.
In this article, you will learn how to effectively harness the cin
object to streamline input operations in C++. You'll explore basic input techniques, handling different data types, and managing input errors to ensure robust data capture in your applications.
Basic Usage of cin
Capturing Integer Data
Prompt the user to enter an integer.
Use
cin
to read the integer input and store it in a variable.cpp#include <iostream> using namespace std; int main() { int userAge; cout << "Enter your age: "; cin >> userAge; cout << "Your age is: " << userAge << endl; return 0; }
This code snippet prompts the user to enter their age, captures it using
cin
, and displays the input back to the user.
Reading Multiple Variables
Understand that
cin
can read multiple inputs separated by spaces.Perform a task that involves capturing different data types.
cpp#include <iostream> using namespace std; int main() { int age; double weight; cout << "Enter your age and weight: "; cin >> age >> weight; cout << "Age: " << age << ", Weight: " << weight << "kg" << endl; return 0; }
This snippet demonstrates how
cin
manages to read an integer and a double input consecutively. The inputs should be provided in the same line, separated by space.
Error Handling in cin
Checking Input Failure
Recognize the need for error checking during input operations.
Use
cin.fail()
to detect input errors.cpp#include <iostream> using namespace std; int main() { int userInput; cout << "Enter an integer: "; cin >> userInput; if (cin.fail()) { cin.clear(); // Clear error state cin.ignore(numeric_limits<streamsize>::max(), '\n'); // Skip bad input cout << "Invalid input, please enter an integer." << endl; } else { cout << "You entered: " << userInput << endl; } return 0; }
This code checks if the input operation failed (e.g., when a non-integer is entered) and handles the error by clearing the error state of
cin
and ignoring the rest of the current input line.
Conclusion
Mastering the use of the cin
object in C++ enhances your ability to write interactive applications that can dynamically process user input. From simple data captures to handling erroneous entries, cin
offers a robust framework for data entry in console-based applications. By implementing the techniques discussed, your applications can effectively interact with users, ensuring accurate and efficient data capture crucial for application functionality. Thus, utilize cin
to its fullest to elevate the user interaction experience in your C++ applications.
No comments yet.