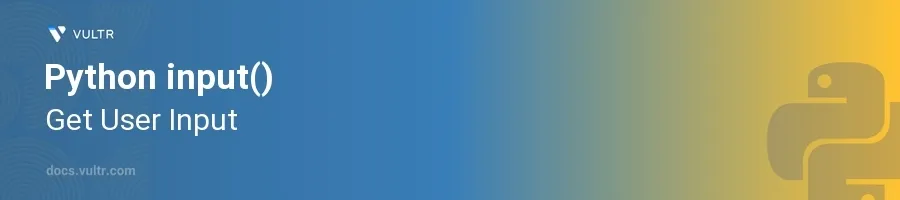
Introduction
The input()
function in Python is an essential tool for interactive programs that require user participation. By capturing user input, you can create more dynamic and responsive applications. Whether it's collecting a user's name, receiving numerical data, or querying specific choices, input()
allows for real-time interaction between the program and the user.
In this article, you will learn how to effectively utilize the input()
function to retrieve various types of user input in Python. Explore practical examples that demonstrate the versatility of this function in different scenarios, improving how you handle user interactions in your programs.
Retrieving Basic Text Input
Prompt for User's Name
Display a message asking the user for their name.
Use the
input()
function to collect the response.pythonname = input("Enter your name: ") print(f"Hello, {name}!")
This code snippet prompts the user to enter their name. The
input()
function pauses the program's execution and waits for the user to type something and press enter. Once the user does so, the function returns the input as a string.
Collect Multiple Inputs
Use multiple
input()
calls to gather different pieces of data.Combine these inputs in a meaningful way in the program.
pythonfirst_name = input("Enter your first name: ") last_name = input("Enter your last name: ") print(f"Your full name is {first_name} {last_name}.")
This example collects the user's first and last names through two separate input calls and then combines them to display the full name.
Handling Numeric Input
Convert Input to Integer
Prompt the user to input a number.
Convert the string input to an integer using
int()
.pythonage = input("Enter your age: ") age = int(age) print(f"You are {age} years old.")
Here, after receiving numeric input as a string, it is converted to an integer type to be used for numerical operations or conditional logic within the program.
Simple Arithmetic Calculator
Get two numbers from the user.
Perform arithmetic operations based on these inputs.
pythonnum1 = int(input("Enter the first number: ")) num2 = int(input("Enter the second number: ")) sum = num1 + num2 print(f"The sum of {num1} and {num2} is {sum}.")
By converting input values with
int()
, you can use these for arithmetic operations like addition. This snippet calculates and displays the sum of two numbers.
Using input() with Conditional Logic
User Choice in Program Flow
Offer the user choices that direct the flow of the program.
Use user input to determine the path of execution.
pythonchoice = input("Would you like to continue? Yes/No: ").lower() if choice == 'yes': print("Continuing the program...") else: print("Exiting the program. Goodbye!")
Depending on the user's choice, this script either continues its execution or terminates. Here,
input()
plays a critical role in decision-making processes within the program.
Conclusion
The input()
function in Python is a fundamental tool for obtaining user feedback, making scripts interactive and flexible. From collecting strings and converting inputs into numerical values to integrating user responses in program logic, mastering input()
enhances your ability to create engaging and user-oriented applications. Employ the examples and techniques discussed to enhance your interaction designs in Python applications.
No comments yet.