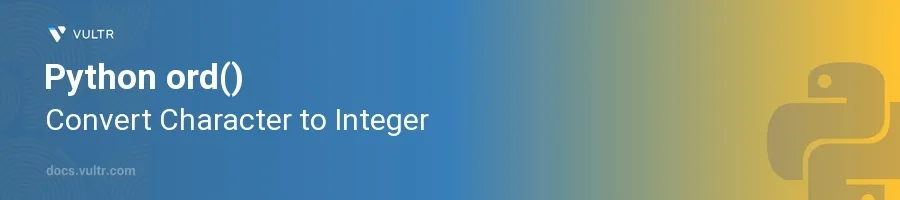
Introduction
The ord()
function in Python is essential for converting a single Unicode character into its corresponding Unicode point integer. This function plays a significant role in various applications, such as cryptographic algorithms, data parsing, and encoding processes where character manipulation at the integer level is required.
In this article, you will learn how to effectively use the ord()
function in Python to handle character-to-integer conversions. Understand its behavior with different types of characters and explore some practical scenarios where ord()
can be particularly useful.
Basics of ord() Function
Convert Basic English Characters
Choose a basic English character.
Pass the character as an argument to the
ord()
function.pythonchar = 'A' unicode_point = ord(char) print(unicode_point)
This code snippet will print the Unicode point of the character 'A', which is
65
. Theord()
function simply converts the character to its corresponding integer, based on the Unicode standard.
Handle Non-English Characters
Select a non-English text character.
Use the
ord()
function to convert this character to its Unicode integer.pythonchar = 'é' unicode_point = ord(char) print(unicode_point)
In this example, 'é' is converted into
233
. Non-English characters, including those with diacritical marks, have unique Unicode points whichord()
can successfully retrieve.
Advanced Usage Scenarios
Using ord() in Cryptographic Conditions
Integrate
ord()
into a basic cryptographic function where each letter is shifted by a set number of places in the Unicode table.Apply the function to encrypt a string.
pythondef encrypt(text, shift): encrypted_text = "" for char in text: shifted = ord(char) + shift encrypted_text += chr(shifted) return encrypted_text sample_text = 'hello' encrypted = encrypt(sample_text, 2) print(encrypted)
The
encrypt
function shifts each character in the input text by 2 Unicode points to create a simple encrypted string. This example reveals the straightforward integration of theord()
function in data encryption processes.
Interaction with Other Functions
Combine
ord()
withmap()
to convert a whole string of characters to their respective Unicode integers.Print the list of Unicode integers.
pythonword = 'Python' unicode_points = list(map(ord, word)) print(unicode_points)
This code snippet will output the list of Unicode integers corresponding to each character in "Python". Using
map()
withord()
provides an efficient way to handle multiple conversions simultaneously.
Conclusion
The ord()
function is a powerful and simple tool in Python for converting characters into their corresponding Unicode integers. This function is invaluable for operations that require interaction with characters at their fundamental integer level, such as in encoding mechanisms, data transformation, and cryptographic applications. By mastering ord()
, you can perform a wide range of tasks, from simple data manipulation to complex encryption algorithms, ensuring accurate and efficient data processing across different applications.
No comments yet.