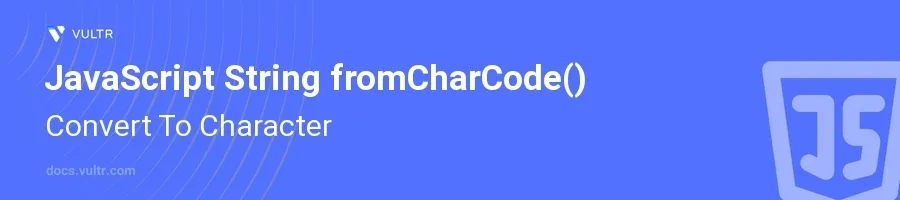
Introduction
The JavaScript method String.fromCharCode()
is a powerful built-in function that converts Unicode values into characters. This tool is indispensable when you need to handle and manipulate character encoding during data processing or when displaying text in web applications.
In this article, you will learn how to use the String.fromCharCode()
method effectively. Explore its basic usage for converting single or multiple Unicode values to characters, and gain insights into practical applications such as generating random strings and resolving encoding issues.
Basic Usage of fromCharCode()
Convert Single Unicode Value to Character
Identify the Unicode value of the character you want to convert.
Use
String.fromCharCode()
to get the corresponding character.javascriptvar char = String.fromCharCode(97); console.log(char);
This code converts the Unicode value
97
to the character'a'
. The method returns 'a', which is the lowercase letter 'a' in the ASCII character set.
Convert Multiple Unicode Values to Characters
Store multiple Unicode values in an array or series of variables.
Apply
String.fromCharCode()
with multiple arguments to generate a string.javascriptvar string = String.fromCharCode(72, 101, 108, 108, 111); console.log(string);
In this snippet, the Unicode values
72
,101
,108
,108
, and111
correspond to 'H', 'e', 'l', 'l', and 'o' respectively, combining to form the word "Hello".
Practical Applications of fromCharCode()
Generate a Random Character String
Use JavaScript's
Math.random()
along withMath.floor()
to generate random Unicode values.Convert these values to characters using
String.fromCharCode()
.javascriptfunction generateRandomString(length) { var randomString = ''; for (var i = 0; i < length; i++) { var randomChar = String.fromCharCode(Math.floor(Math.random() * 26) + 97); randomString += randomChar; } return randomString; } console.log(generateRandomString(5));
This function generates a random string of lowercase letters. It repeatedly picks a random number between 97 and 122 (inclusive), corresponding to the Unicode values for 'a' to 'z', and converts each to a character to form a string.
Decode Unicode Escape Sequences
Understand the representations of Unicode escape sequences.
Use
String.fromCharCode()
to turn these sequences into human-readable characters.javascriptvar unicodeString = '\\u0041\\u0042\\u0043'; var decodedString = unicodeString.split('\\u').slice(1).map(u => String.fromCharCode(parseInt(u, 16))).join(''); console.log(decodedString);
This snippet processes a string containing Unicode escape sequences for 'A', 'B', 'C', decodes them using
String.fromCharCode()
, and outputs the string "ABC". The Unicode hexadecimal values are parsed to integers which are then converted to their respective characters.
Conclusion
The String.fromCharCode()
method in JavaScript simplifies the conversion of Unicode values to characters, enhancing control over text manipulation and encoding in your web applications. Its straightforward syntax and flexibility make it a valuable tool for a variety of tasks including data encoding, decoding, and generating random text. Utilize this method to handle character-related operations efficiently, ensuring your applications accurately interpret and display textual data.
No comments yet.