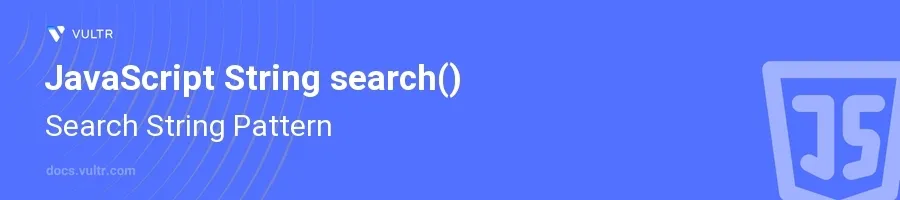
Introduction
The search()
method in JavaScript is a versatile tool used to search for a pattern within a string, returning the position of the match. This method proves particularly useful for quickly locating substrings or patterns described by regular expressions within larger text bodies, enhancing text processing capabilities in JavaScript applications.
In this article, you will learn how to effectively use the search()
method to find patterns in strings. Explore scenarios involving plain text searches and more complex pattern matching with regular expressions, enhancing your JavaScript coding efficiency and capability in handling string operations.
Basic Usage of search()
Searching for a Simple Text
Define the string in which the search will be performed.
Use the
search()
method with a direct text query.javascriptlet myString = "Hello, welcome to the programming world."; let position = myString.search("welcome"); console.log(position);
This code snippet searches for the word
"welcome"
inmyString
. Thesearch()
method returns the index of the first occurrence, which, in this case, is7
.
Case Sensitivity in search()
Recognize that
search()
is sensitive to text case.Compare outcomes using different cases in the search pattern.
javascriptlet anotherString = "Hello, Welcome to the programming world."; let caseSensitivePosition = anotherString.search("welcome"); console.log(caseSensitivePosition);
In this example, even though the word
"Welcome"
is present,search()
looks for"welcome"
with a lowercase "w" and consequently does not find a match, returning-1
.
Using Regular Expressions
Search with Basic Regex Patterns
Use regular expressions to create more flexible search criteria.
Implement a regex pattern within the search method.
javascriptlet regexString = "JavaScript, HTML, CSS, JavaScript"; let regexPosition = regexString.search(/html/i); console.log(regexPosition);
Here, the regular expression
/html/i
includes thei
flag for case insensitivity. The search method finds the first occurrence of"HTML"
and returns its position, demonstrating the capability of regular expressions to enhance search flexibility.
Advanced Regex Features
Leverage advanced regex functionalities to refine search patterns.
Use special regex symbols and qualifiers to craft precise searches.
javascriptlet complexString = "Mr. Blue has a blue house"; let complexSearch = complexString.search(/\bblue\b/i); console.log(complexSearch);
In this snippet, the pattern includes
\b
to ensure"blue"
is checked as a whole word, not as part of another word. Thei
flag continues to ignore case sensitivity. This returns the position of"blue"
in the context of a whole word match.
Conclusion
The search()
function in JavaScript is crucial for pattern identification in strings, particularly when parsing and manipulating text. Integrating regular expressions with the search()
method greatly expands its utility, allowing for more sophisticated search operations. By understanding and applying the examples and techniques discussed, you have enriched your toolkit for tackling string-related tasks in your JavaScript applications, ensuring both flexibility and efficiency in your programming endeavors.
No comments yet.