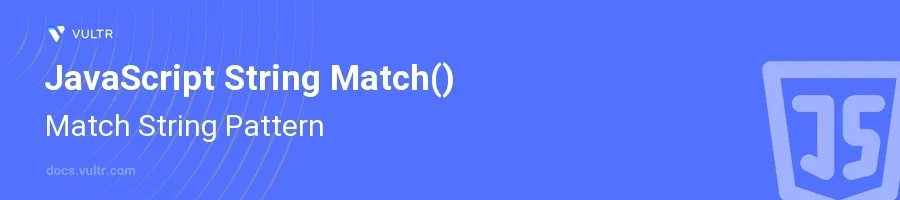
Introduction
The match()
method in JavaScript is used to retrieve the results of matching a string against a regular expression. This method is very handy when you need to extract specific parts of strings based on patterns, or check if a string contains a certain substring satisfying a pattern.
In this article, you will learn how to utilize the match()
method in JavaScript through various examples. Understand how to apply this method with both string literals and dynamically constructed regular expressions to match patterns effectively in your JavaScript projects.
Using match() to Extract Data
Extracting Words from a String
Define a string with multiple words.
Use a regular expression to match all word characters.
javascriptconst text = "Hello world, welcome to the universe."; const words = text.match(/\w+/g); console.log(words);
This code snippet uses the
match()
function to find all word characters repeatedly (\w+
) in the given text. Theg
flag in the regular expression stands for "global," which means it finds all matches rather than stopping at the first match.
Finding Specific Patterns
Consider a scenario where you need to find dates formatted as
dd/mm/yyyy
.Use
match()
with a suitable regular expression.javascriptconst diaryEntry = "I visited the museum on 15/08/2023."; const datePattern = diaryEntry.match(/\d{2}\/\d{2}\/\d{4}/); console.log(datePattern);
In this example, the regular expression looks for patterns that match two digits, followed by a slash, another two digits, another slash, and then four digits, which is a common date format. The returned match is
'15/08/2023'
.
Case Sensitivity in match()
Ignoring Case While Matching
Suppose you want to match a word in a case-insensitive way.
Use the regular expression flag
i
.javascriptconst greeting = "Hello World"; const result = greeting.match(/world/i); console.log(result);
By using the
i
flag (insensitive), this code ignores the case of the characters when matching the string "world" with "World".
Using match() for Validation
Email Validation Example
Define a regular expression for a simple email pattern.
Check if a string is a valid email using the
match()
method.javascriptconst input = "user@example.com"; const isValidEmail = input.match(/^\w+@\w+\.\w+$/); console.log(isValidEmail ? "Valid Email" : "Invalid Email");
This regular expression checks whether the string starts with one or more word characters, followed by an
@
, more word characters, a dot, and ends with one or more word characters, thus validating a basic email format.
Conclusion
The match()
method in JavaScript is an essential function for string manipulation, allowing programmers to efficiently extract data, perform pattern-matching, and validate inputs against specific formats. Utilize this method in various contexts where pattern recognition is crucial. Adapt and extend the examples provided to accommodate different scenarios in your web applications, ensuring robust data handling and user input validation.
No comments yet.