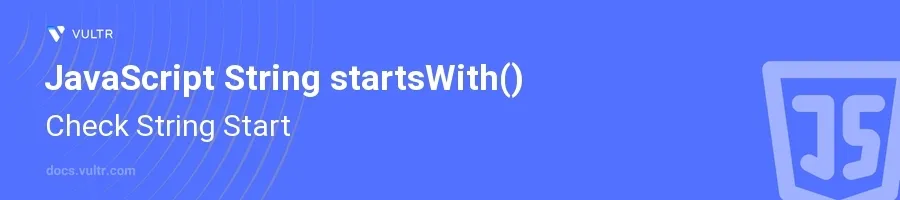
Introduction
The startsWith()
method in JavaScript is essential for checking if a given string begins with a specified substring. This functionality is widely used across web development tasks, including form validation, data parsing, and conditionally rendering components based on textual data.
In this article, you will learn how to effectively harness the startsWith()
method in JavaScript. Explore practical examples to understand how you can implement this method to check the starts of strings in various programming contexts.
Understanding startsWith() Method
Basic Usage of startsWith
- Get familiar with the syntax of
startsWith()
: JavaScript providesstartsWith()
as a method of the String prototype, allowing you to check prefixes in strings straightforwardly. - Notice the parameters it takes:
- The substring to search for at the start of the string.
- An optional position index that defaults to 0, where the method should begin checking.
Check the Beginning of a String
Define a string.
Use
startsWith()
to determine if it begins with a specific substring.javascriptconst phrase = "Hello world!"; const result = phrase.startsWith("Hello"); console.log(result);
This snippet outputs
true
because "Hello world!" indeed starts with "Hello".
Utilizing the Position Parameter
Understand that you can specify a starting position in the string: This allows checking if the substring appears not just at the beginning but at any specified position.
Apply this feature by providing a second argument to
startsWith()
.javascriptconst sentence = "JavaScript is versatile!"; const check = sentence.startsWith("is", 11); console.log(check);
Here,
startsWith()
checks if "JavaScript is versatile!" has the substring "is" starting from index 11. It returnstrue
since "is" starts at that position.
Working with Dynamic Content
Case Sensitivity in startsWith()
Remember that
startsWith()
is case-sensitive: It's crucial to consider this when dealing with user inputs or data from various sources.Implement a basic case-insensitive check using
toLowerCase()
ortoUpperCase()
.javascriptconst input = "Today is sunny."; const search = "today"; const caseInsensitiveCheck = input.toLowerCase().startsWith(search.toLowerCase()); console.log(caseInsensitiveCheck);
This adjustment ensures the comparison disregards case differences, thus, "Today is sunny." starts with "today" under a case-insensitive logic.
Combining startsWith() in Conditions
Combine
startsWith()
with other string methods to build complex conditions.Create multifaceted checks utilizing
startsWith()
.javascriptconst userCommand = "play video"; if (userCommand.startsWith("play") && userCommand.includes("video")) { console.log("Playing video..."); }
It checks if the
userCommand
starts with "play" and includes "video" anywhere in the string, hence executing a more complex logic based on user input.
Conclusion
The startsWith()
method in JavaScript provides a straightforward, efficient way to evaluate whether a string starts with a specified substring. From performing simple checks to integrating it into complex conditional statements, this method significantly contributes to string manipulation tasks in JavaScript. By mastering startsWith()
, you enhance your ability to handle text-based data effectively, ensuring your applications respond dynamically and appropriately to various inputs.
No comments yet.