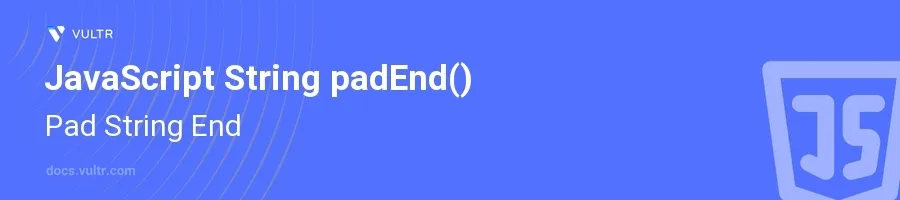
Introduction
The padEnd()
method in JavaScript is crucial for working with strings, especially when you need to ensure that a string reaches a certain length by padding it with characters at the end. This method fills the current string with a given string (repeated, if necessary) until the resulting string reaches the provided length. It's incredibly useful for formatting output in applications, aligning text, or preparing data for consistent storage and retrieval.
In this article, you will learn how to efficiently use the padEnd()
method in JavaScript. Discover the method's syntax, explore several real-world examples of how to pad strings effectively, and understand the behavior when dealing with different parameters and edge cases.
Understanding padEnd() Syntax and Parameters
Before diving into examples, grasp the basics of padEnd()
. Here’s how to utilize this method:
- Identify the target string you wish to pad.
- Determine the total length of the output string after padding.
- Choose the string you want to use for padding (optional).
The syntax of padEnd()
is relatively simple:
let paddedString = originalString.padEnd(targetLength[, padString]);
originalString
is the string you want to pad.targetLength
is the desired length of the string after padding.padString
is the string used to fill the gap. If not specified, the default is a space (' ').
By not providing a padString
, padEnd()
pads the string with spaces:
let text = "Hello";
console.log(text.padEnd(10)); // Outputs: "Hello "
Here, the string "Hello" is padded with five spaces to achieve a length of 10.
Practical Use Cases of padEnd()
Formatting Table Output
Assume you need to neatly format rows in a console output where each column should align.
Use
padEnd()
to ensure each string in the columns is of the same length.javascriptlet names = ["Alice", "Bob", "Christina"]; let paddedNames = names.map(name => name.padEnd(10, ' ')); paddedNames.forEach(name => console.log(name));
This example ensures each name in the list now aligns right in a 10-character width, ideal for table formatting.
Setting Up Fixed-Length Record
When storing data in a format with fixed-length records, padding strings to a specific length becomes critical.
Apply
padEnd()
to maintain uniform record lengths.javascriptlet record = "40213"; console.log(record.padEnd(10, '0')); // Outputs: "4021300000"
Padding the record "40213" with zeros up to 10 characters is handy in contexts where data must fit a predefined format.
Managing Default Values
Often in user interfaces, you need to display a default value if a variable is undefined or empty.
Use
padEnd()
to handle cases where the content might be shorter than expected.javascriptlet content = ""; console.log(content.padEnd(10, 'NA')); // Outputs: "NANANANANA"
In this scenario, if content is missing, the field is padded with "NA" repeating until the field reaches the designated length.
Conclusion
The padEnd()
function in JavaScript is a powerful tool for aligning text, ensuring uniform data storage, and handling formatting in a visually pleasing manner. By incorporating this method into your code, you enhance the clarity and professionalism of your output, catering to both utility and aesthetic needs. As demonstrated with various examples ranging from simple to complex, padEnd()
is adaptable to meet diverse text manipulation needs and scenarios. Adopt the techniques discussed to ensure your strings always meet the necessary specifications for length and appearance.
No comments yet.