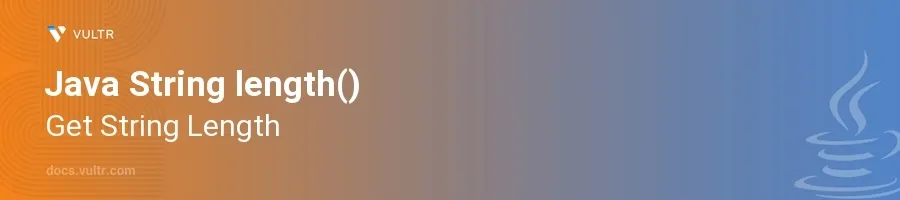
Introduction
The length()
method in Java is a simple yet essential tool in the programming toolkit. It is part of the String
class in Java and is used to determine the number of characters contained in a string. This method is pivotal when you need to evaluate or manipulate strings, especially in data validation, formatting, and processing operations.
In this article, you will learn how to effectively utilize the length()
method in various programming scenarios. Explore techniques to harness this method for tasks such as validating user input, handling text data efficiently, and implementing logical conditions based on string length.
Understanding the length() Method
Basic Usage of length()
Create a string variable.
Apply the
length()
method to determine the number of characters in the string.javaString example = "Hello, world!"; int lengthOfString = example.length(); System.out.println("Length of the string: " + lengthOfString);
This code snippet creates a string containing "Hello, world!" and uses
length()
to find out its length. The output will be15
, since there are 15 characters, including punctuation and spaces.
Checking Empty Strings
Consider situations where validating an empty string is necessary, like in form submissions or fields where data is required.
Utilize the
length()
method to check if a string is empty.javaString emptyString = ""; boolean isEmpty = emptyString.length() == 0; System.out.println("Is string empty? " + isEmpty);
Here,
isEmpty
will betrue
because the length ofemptyString
is0
. This reflects that the string contains no characters.
Advanced Uses of length()
Conditional Processing Based on String Length
Use string length to conditionally process data.
Ensure that logical operations are only performed when string lengths meet certain criteria.
javaString userInput = "example"; if (userInput.length() > 5) { System.out.println("Input accepted."); } else { System.out.println("Input is too short. Must be at least 6 characters."); }
This example demonstrates a conditional where the input must be at least 6 characters long to be accepted. This is a common validation requirement in user forms.
Looping with length() in Control Structures
Use the
length()
method as a control parameter in loop structures.Preface actions or validations that depend on a character-by-character analysis of the string.
javaString phrase = "Iterate this"; for (int i = 0; i < phrase.length(); i++) { char character = phrase.charAt(i); System.out.println("Character at position " + i + ": " + character); }
The
for
loop iterates over each character in the stringphrase
by usinglength()
to limit the loop range. This method allows access to each character sequentially, which is useful for parsing or modifying strings.
Conclusion
The length()
method in Java's String class is critically useful for controlling and manipulating text-based data, providing fundamental support for string operations like validations, conditional processing, and iterative control. By mastering this method, you enhance your capability to handle strings effectively in your Java projects. Regardless of the complexity of your data or the requirements of your tasks, knowing how to apply the length()
method ensures that your text processing logic is efficient and robust.
No comments yet.