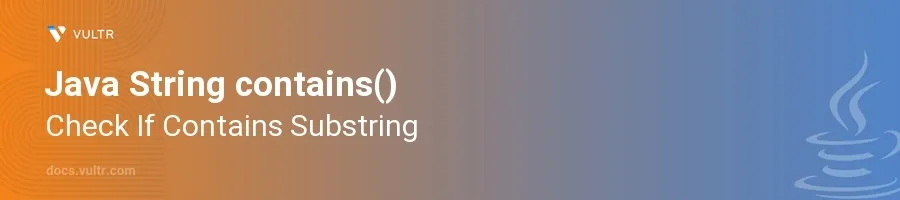
Introduction
The contains()
method in Java is a crucial function for string manipulation, used extensively to determine whether a particular substring exists within another string. Ideal for validation, search filters, or processing textual data, this method enhances functionality and efficiency in many Java applications.
In this article, you will learn how to effectively use the String.contains()
method in Java. Explore its basic usage for substring checks, its application with conditional statements for dynamic searching, and understand common pitfalls to avoid for optimal code performance.
Basic Usage of contains()
Check for the Presence of a Substring
Initialize a string which will be searched.
Use the
contains()
method to verify the presence of a substring.javaString mainString = "Hello, world!"; boolean result = mainString.contains("world"); System.out.println(result);
This code prints
true
because "world" is a substring ofmainString
. Thecontains()
method is case-sensitive and hence, accuracy in casing is crucial.
Handling Case Sensitivity
Convert both the main string and the substring to the same case.
Apply the
contains()
method.javaString mainString = "Hello, World!"; String subStringToCheck = "world"; boolean result = mainString.toLowerCase().contains(subStringToCheck.toLowerCase()); System.out.println(result);
By converting both strings to lower case, the method reliably checks for the presence of "world" irrespective of the original casing, which results in
true
.
Dynamic Substring Search in Applications
Using contains() in Conditional Statements
Use
contains()
within anif
orelse
condition to execute code based on substring presence.javaString mainString = "Java programming is fun!"; if (mainString.contains("programming")) { System.out.println("The string talks about programming!"); } else { System.out.println("No mention of programming here."); }
Since "programming" is found in
mainString
, the output will be "The string talks about programming!". This demonstrates howcontains()
can drive logic flow in programs.
Common Pitfalls and Considerations
Null References
Ensure that the string variable is not
null
before invokingcontains()
.javaString mainString = null; try { boolean result = mainString.contains("text"); System.out.println(result); } catch (NullPointerException e) { System.out.println("String reference is null."); }
Accessing a method on a
null
reference leads to aNullPointerException
. Always check or handle potentialnull
values gracefully within your code.
Performance Implications
- Recognize that
contains()
might be slow for very long strings or within tight loops. - Optimize by considering alternative methods like regex or specialized libraries if performance under scale is crucial.
Conclusion
The contains()
method in the Java String
class is an indispensable tool for checking the presence of substrings within another string. It offers a straightforward, readable approach to string search operations, essential in many programming contexts. However, remember to consider case sensitivity and null safety to avoid common errors. By integrating this function effectively into your Java applications, you achieve more robust and reliable text processing capabilities.
No comments yet.