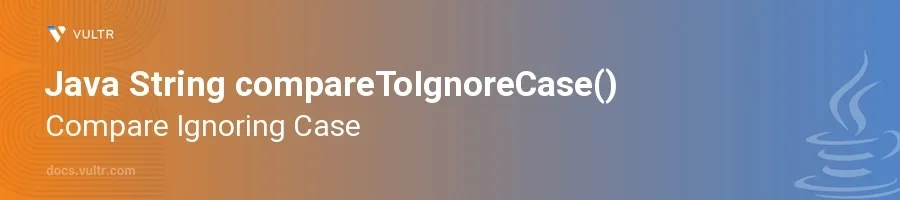
Introduction
The compareToIgnoreCase()
method in Java is pivotal for comparing two strings lexicographically, ignoring case considerations. It plays a crucial role especially in scenarios where you want string comparisons to be case-insensitive, such as sorting names in an address book or searching in a case-insensitive manner.
In this article, you will learn how to use the compareToIgnoreCase()
method effectively in Java. Examples will demonstrate basic string comparisons, handling null values, integrating this method into user-defined object sorting, and practical applications in case-insensitive data retrieval.
Basic Usage of compareToIgnoreCase()
Comparing Two Strings
Declare and initialize two strings for comparison.
Use the
compareToIgnoreCase()
method and store the result.javaString str1 = "Hello"; String str2 = "hello"; int result = str1.compareToIgnoreCase(str2); System.out.println(result);
In this code,
str1.compareToIgnoreCase(str2)
returns0
indicating that the strings are equal disregarding the case.
Checking for Ordering
Compare strings to find out their order ignoring case.
Print the output based on the comparison.
javaString str1 = "apple"; String str2 = "Banana"; int orderResult = str1.compareToIgnoreCase(str2); if (orderResult < 0) { System.out.println("apple is lexicographically smaller than Banana."); } else if (orderResult > 0) { System.out.println("apple is lexicographically greater than Banana."); } else { System.out.println("Strings are equal."); }
Here, although 'B' comes before 'a' in capital case, ignoring cases, "apple" is lexicographically smaller.
Handling Null Values
Secure Comparison Including Null Checks
Implement a helper method to safely compare two strings considering possible null values.
Use the devised method to compare strings.
javapublic static int safeCompareToIgnoreCase(String str1, String str2) { if (str1 == null ^ str2 == null) { return (str1 == null) ? -1 : 1; } if (str1 == null && str2 == null) { return 0; } return str1.compareToIgnoreCase(str2); }
This method handles possible null inputs safely, preventing
NullPointerException
. If either string is null, it assigns a lesser value to it to maintain order consistency.
Integrating with Custom Sorting
Using compareToIgnoreCase() in Custom Objects
Define a class with a
String
field.Implement
Comparable
to sort objects by the string field, ignoring case.Utilize
compareToIgnoreCase()
in thecompareTo
method.javaclass Person implements Comparable<Person> { private String name; Person(String name) { this.name = name; } public int compareTo(Person other) { return this.name.compareToIgnoreCase(other.name); } }
Sorting a list of
Person
objects now respects a case-insensitive order of names.
Conclusion
The compareToIgnoreCase()
method in Java provides a straightforward, powerful tool for case-insensitive string comparisons. It enhances functionality in sorting and searching operations where case sensitivity is irrelevant. By leveraging this method, you facilitate robust data handling and operations in various Java applications. The examples provided guide you through utilizing this method in multiple scenarios effectively, ensuring clarity and efficiency in code dealing with textual data comparisons.
No comments yet.