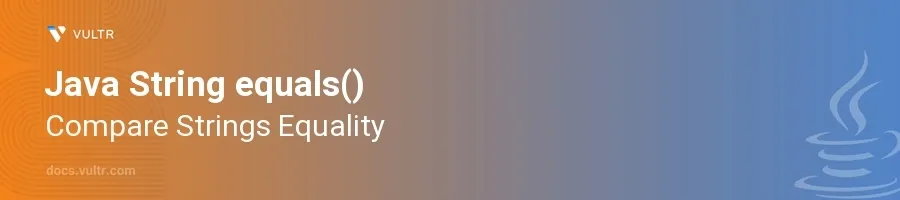
Introduction
Comparing strings is a fundamental task in Java, often necessary to ensure data integrity, validate user inputs, manage control flow, and more. The equals()
method in the String
class is the primary tool for comparing the text of two strings, ensuring character-by-character equality without considering object identity in memory. This aspect is crucial because different string objects can contain identical sequences of characters.
In this article, you will learn how to utilize the String.equals()
method in Java efficiently. Explore how to check string equality under various scenarios, handle potential pitfalls, and employ best practices to make your code robust and maintainable. This guide also includes examples that illustrate proper usage in real-world applications for both beginners and experienced programmers.
Basic Usage of String.equals()
Comparing Two Strings
Assign values to two string variables.
Utilize the
equals()
method to compare these strings.javaString str1 = "hello"; String str2 = "hello"; boolean isEqual = str1.equals(str2); System.out.println(isEqual);
Here,
str1
andstr2
are initialized with the same string value "hello". Theequals()
method checks ifstr1
andstr2
have the same characters in the same order, returningtrue
because they are identical.
Case Sensitivity
Note that string comparison using
equals()
is case-sensitive.Create strings with different capitalization.
Compare these using the
equals()
method.javaString str1 = "Hello"; String str2 = "hello"; boolean isEqual = str1.equals(str2); System.out.println(isEqual);
In this example, although
str1
andstr2
contain similar characters, they differ in case. Consequently, theequals()
method returnsfalse
.
Handling null Values
Avoiding NullPointerException
Understand that invoking
equals()
on anull
reference will throw aNullPointerException
.Perform a null check before using
equals()
for comparison.Optionally use Java's Objects class for a null-safe comparison.
javaString str1 = null; String str2 = "java"; boolean isEqual = (str1 != null && str1.equals(str2)); System.out.println(isEqual); // Using Objects class isEqual = java.util.Objects.equals(str1, str2); System.out.println(isEqual);
In the first method, a direct null check guards against
NullPointerException
by ensuringstr1
is notnull
before comparing. The second approach utilizes theObjects.equals()
method, which is null-safe and eliminates the need for explicit null checks.
Real-world Application Scenarios
User Authentication
Use
equals()
for password checks in a login system.Securely compare entered password with the stored password.
javaString storedPassword = "securePassword123"; String inputPassword = getPasswordFromUserInput(); // Assume this method retrieves the password a user enters if (storedPassword.equals(inputPassword)) { System.out.println("Login successful."); } else { System.out.println("Incorrect password."); }
This example demonstrates how
equals()
is used to validate user logins. It compares the password entered by the user against the stored, correct password. Accurate string matching is critical to ensure security and access control in applications.
Conclusion
The String.equals()
function in Java is an essential method for string comparison, pivotal in many programming tasks, such as data validation, conditional logic, and user authentication. Its ability to discern character sequences robustly—while giving a simple interface for equality checking—makes it indispensable. By incorporating these best practices and using equals()
correctly, empower your Java applications with reliable, error-free string comparison mechanisms, enhancing both security and functionality. Ensure to always consider potential null values and remember the case sensitivity in comparisons to avoid bugs and ensure accurate logic flows in your code.
No comments yet.