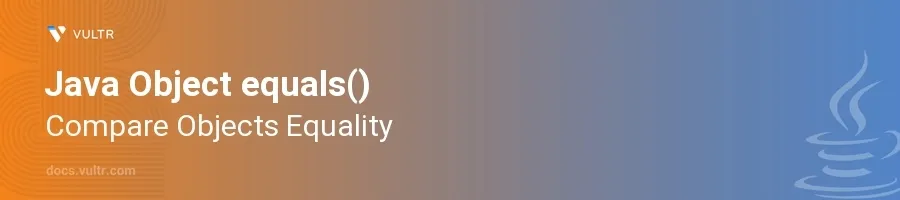
Introduction
The equals()
method in Java is a fundamental mechanism for comparing two objects for equality. It plays a crucial role in collections, such as HashSet
and HashMap
, that rely on equality checks to function correctly. Understanding how to implement and use the equals()
method properly is essential for Java developers to ensure that their classes interact correctly with Java's collection framework.
In this article, you will learn how to correctly utilize the equals()
method to compare object equality in Java. Explore different scenarios and customization of the equals()
method that suit various class structure needs, enhancing the robustness of your Java applications.
Understanding the equals() Method
Basic Usage of equals()
Know that by default, the
equals()
method in Java compares the memory addresses of objects.Override the
equals()
method to suit the specific needs of the class.javaclass Person { private String name; private int age; Person(String name, int age) { this.name = name; this.age = age; } @Override public boolean equals(Object obj) { if (this == obj) return true; if (obj == null || getClass() != obj.getClass()) return false; Person person = (Person) obj; return age == person.age && name.equals(person.name); } }
This code snippet demonstrates how to override the
equals()
method for aPerson
class, where equality is based on both name and age attributes. The method first checks if the object reference is the same, then checks for null and class match, and finally compares individual fields for equality.
Considerations for Implementing equals()
- Ensure symmetry: For any non-null values
x
andy
,x.equals(y)
should returntrue
if and only ify.equals(x)
returnstrue
. - Ensure transitivity: For any non-null values
x
,y
, andz
, ifx.equals(y)
returnstrue
andy.equals(z)
returnstrue
, thenx.equals(z)
should also returntrue
. - Ensure consistency: Multiple invocations of
x.equals(y)
consistently returntrue
or consistently returnfalse
, provided no information used inequals()
comparisons is modified. - For any non-null reference value
x
,x.equals(null)
should returnfalse
.
Practical Applications of equals()
Using equals() in Collections
Understand the importance of overriding
equals()
when dealing with collections likeArrayList
,HashSet
, orHashMap
.Realize that collections use the
equals()
method to determine element uniqueness and retrieve elements.javaArrayList<Person> list = new ArrayList<>(); list.add(new Person("John", 25)); list.add(new Person("Alice", 30)); boolean contains = list.contains(new Person("John", 25)); System.out.println("List contains John: " + contains);
This example shows the usage of overridden
equals()
in collections to check for the presence of an object. Becauseequals()
has been customized,new Person("John", 25)
is considered equal to an existing object in the list.
Conclusion
The equals()
method in Java is a powerful tool for determining object equality with precision. Proper implementation of this method is crucial for classes whose instances might be stored in various collections, such as lists or maps. Mastering the customization and utilization of the equals()
method ensures that your Java applications can handle complex scenarios involving object equality, enhancing both performance and reliability. Follow the guidelines and examples discussed to implement this method effectively in your Java classes.
No comments yet.