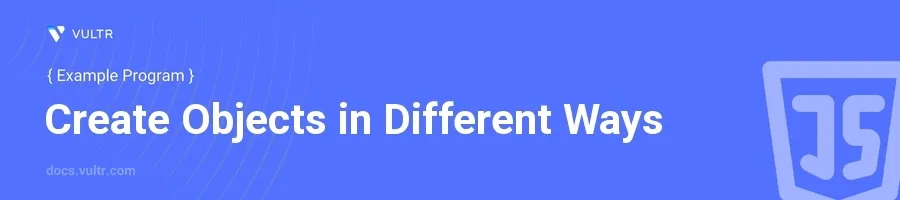
Introduction
JavaScript objects are versatile and flexible constructs used extensively in programming for organizing data and behavior. They can be created in various ways, each with its own advantages and use cases. Understanding these different methods is crucial for effective coding, especially when dealing with complex data structures or API responses.
In this article, you will learn how to create JavaScript objects using multiple techniques. Explore each method with practical examples to understand when and why to use them in your development projects.
Creating Objects Using Object Literals
Simple Object with Properties
Define an object using curly braces
{}
.Add properties and their values within the braces.
javascriptconst person = { name: "John Doe", age: 30 }; console.log(person);
This code snippet creates an object
person
with two properties:name
andage
. Theconsole.log()
function is used to display the object in the console.
Object with Methods
Extend the object with functions that perform actions related to the object.
Define these functions within the object literal.
javascriptconst person = { name: "Jane Doe", age: 28, greet: function() { console.log("Hello, my name is " + this.name); } }; person.greet();
Here,
greet
is a method ofperson
which, when called, prints a greeting message that includes the person's name.
Creating Objects Using Constructor Functions
Defining and Using a Constructor Function
Create a constructor function that specifies an object's properties and methods.
javascriptfunction Person(name, age) { this.name = name; this.age = age; this.greet = function() { console.log("Hello, my name is " + this.name); }; }
Use the
new
keyword to create instances of the object.javascriptconst person1 = new Person("Alice", 24); const person2 = new Person("Bob", 22); person1.greet(); person2.greet();
The constructor function
Person
allows for the creation of multipleperson
objects with the same properties and methods. Each instance is initialized with its own data.
Creating Objects Using the Object.create() Method
Prototype Object as a Template
Define an object that serves as a prototype.
Use
Object.create()
to create new objects inheriting from the prototype.javascriptconst prototypePerson = { greet: function() { console.log("Hello, my name is " + this.name); } }; const person1 = Object.create(prototypePerson); person1.name = "Charlie"; person1.age = 27; person1.greet();
In this example,
person1
is created withprototypePerson
as its prototype. Properties likename
andage
are added later, and thegreet
method is inherited.
Creating Objects Using ES6 Classes
Class Definition and Instantiation
Define a class with properties and methods.
Use the
new
keyword to create instances of the class.javascriptclass Person { constructor(name, age) { this.name = name; this.age = age; } greet() { console.log("Hello, my name is " + this.name); } } const person1 = new Person("Diana", 35); person1.greet();
This method mimics traditional OOP (Object-Oriented Programming) structures in other languages, bringing familiarity and clarity to JavaScript object creation and handling.
Conclusion
Creating JavaScript objects can be approached from several angles, each providing specific benefits depending on the context. Whether using simple object literals for straightforward data objects, constructor functions for more complex instances, the Object.create()
method for differential inheritance, or ES6 classes for a more structured OOP approach, mastering these techniques ensures robust and efficient code. Understanding when to use each can significantly enhance your JavaScript coding projects, making it easier to manage and scale complex applications.
No comments yet.