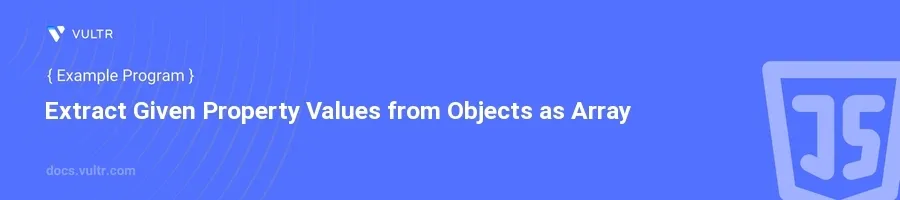
Introduction
When working with JavaScript, one frequently encounters scenarios where it is necessary to extract values from properties of objects and represent them as an array. This process is especially prevalent in data handling and transformation tasks, where objects represent records or entities, and certain operations require just a subset of each object's data.
In this article, you will learn how to extract given property values from an array of objects and return these values as a new array. This knowledge is highly useful for manipulating data structures in JavaScript effectively. Various examples will be provided to demonstrate the practical applications of these techniques.
Extracting Single Property Values
Example with Simple Objects
Extracting values of a single property from an array of objects is a fundamental operation in JavaScript data manipulation.
Consider an array of objects where each object has properties like
id
,name
, andage
.Extract all
name
properties into a new array.javascriptconst people = [ { id: 1, name: "Alice", age: 25 }, { id: 2, name: "Bob", age: 30 }, { id: 3, name: "Charlie", age: 28 } ]; const names = people.map(person => person.name); console.log(names);
This code snippet maps through each object in the
people
array and extracts thename
property, placing it into the new arraynames
. When logged,names
will output:["Alice", "Bob", "Charlie"]
.
Handling Missing Properties
Sometimes not all objects in an array might have the desired property. It's important to handle these cases.
Revise the example to handle cases where the
name
property might be missing from some objects.Use the ternary operator to provide a default value or skip undefined properties.
javascriptconst people = [ { id: 1, name: "Alice", age: 25 }, { id: 2, age: 30 }, { id: 3, name: "Charlie", age: 28 } ]; const names = people.map(person => person.name ? person.name : 'Unknown'); console.log(names);
In this code, the map function checks if
person.name
exists. If it does not, it defaults to 'Unknown'. The resulting array would be:["Alice", "Unknown", "Charlie"]
.
Extracting Multiple Property Values
Concatenating Values from Multiple Properties
Extract values from more than one property and perhaps combine them.
Extract both
name
andage
properties and format them into a string.Collect these strings into a new array.
javascriptconst people = [ { id: 1, name: "Alice", age: 25 }, { id: 2, name: "Bob", age: 30 }, { id: 3, name: "Charlie", age: 28 } ]; const nameAges = people.map(person => `${person.name} is ${person.age} years old`); console.log(nameAges);
This snippet will output an array of strings:
["Alice is 25 years old", "Bob is 30 years old", "Charlie is 28 years old"]
. Each string combines thename
andage
properties of each object.
Using Advanced Filtering and Mapping
Filtering Objects by Property Before Extracting Values
Apply filters based on properties before mapping values.
Filter out objects where the
age
is greater than 25, then extractname
.Produce an array of names satisfying this condition.
javascriptconst people = [ { id: 1, name: "Alice", age: 25 }, { id: 2, name: "Bob", age: 30 }, { id: 3, name: "Charlie", age: 28 } ]; const filteredNames = people.filter(person => person.age > 25).map(person => person.name); console.log(filteredNames);
The
filter
function first selects elements where theage
is greater than 25, and then themap
function extracts just thename
property. The output will be:["Bob", "Charlie"]
.
Conclusion
Mastering the use of the map()
and filter()
functions in JavaScript to extract property values from objects and present them as arrays unlocks a powerful toolkit for data manipulation. Whether dealing with simple scenarios or large datasets requiring complex filtering, these methods provide efficient solutions. Start incorporating these techniques in various programming situations to simplify and enhance data handling in your JavaScript projects.
No comments yet.