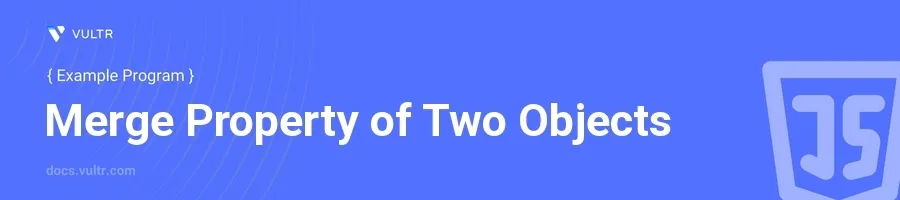
Introduction
Merging properties of two objects in JavaScript is a common task that developers encounter, especially when dealing with application state or configurations. This operation involves taking properties from two different objects and combining them into one object, possibly overriding properties in one object with properties from another if they share the same key.
In this article, you will learn how to merge properties of two objects effectively in JavaScript. Explore various methods including the use of the spread operator, Object.assign()
, and other techniques to handle this task efficiently. Practical examples will guide you through these methods to deepen your understanding and help you apply them in your projects.
Using the Spread Operator
Basic Merging
Define two JavaScript objects with some overlapping properties.
Merge these objects using the spread operator, ensuring properties from the second object overwrite the first if they conflict.
javascriptconst object1 = { name: "Alice", age: 25 }; const object2 = { age: 30, city: "New York" }; const mergedObject = { ...object1, ...object2 }; console.log(mergedObject);
In this code,
object1
andobject2
are merged intomergedObject
. The age property inobject1
is overwritten by the age fromobject2
because it appears later in the merge order.
Avoiding Mutation of Original Objects
Start with the initial objects as defined previously.
Use the spread operator within a new object declaration to ensure the original objects remain unchanged.
javascriptconst mergedObject = { ...object1, ...object2 };
This technique creates a new object entirely, ensuring that neither
object1
norobject2
is modified during the process.
Using Object.assign()
Simple Property Merging
Use
Object.assign()
to combine the properties of two objects into a new object.Note how the properties of the source object (second argument) overwrite those of the target object (first argument).
javascriptconst object1 = { name: "Alice", age: 25 }; const object2 = { age: 30, city: "New York" }; const mergedObject = Object.assign({}, object1, object2); console.log(mergedObject);
The
Object.assign()
function takes an empty object as the first argument to ensure no mutation of existing objects occurs, with subsequent objects being merged into this new empty object.
Deep Copy Concerns
Recognize that both the spread operator and
Object.assign()
perform a shallow copy.Understand implications for nested objects, which remain referenced rather than copied.
javascriptconst object1 = { user: { name: "Alice", age: 25 } }; const object2 = { user: { age: 30 } }; const mergedObject = Object.assign({}, object1, object2); console.log(mergedObject);
This will demonstrate that only the reference to the nested
user
object is copied, not the contents themselves. Any nested properties are not separately merged but wholly replaced.
Custom Function for Deep Merging
Implementing a Deep Merge
Create a function that recursively checks each property and determines whether it needs to merge objects or overwrite primitives.
Utilize recursion to handle nested objects appropriately.
javascriptfunction deepMerge(obj1, obj2) { const result = {}; for (const key in obj1) { if (obj1.hasOwnProperty(key)) { const val1 = obj1[key]; const val2 = obj2[key]; if (typeof val1 === 'object' && val1 !== null && typeof val2 === 'object' && val2 !== null) { result[key] = deepMerge(val1, val2); } else { result[key] = val2 === undefined ? val1 : val2; } } } for (const key in obj2) { if (obj2.hasOwnProperty(key) && result[key] === undefined) { result[key] = obj2[key]; } } return result; } const object1 = { user: { name: "Alice", age: 25 }}; const object2 = { user: { age: 30, city: "New York" }}; const mergedObject = deepMerge(object1, object2); console.log(mergedObject);
This function ensures a true 'deep' merge, where nested objects are combined rather than overwritten. Each property is carefully evaluated to decide how it should be merged, offering a robust solution for more complex object structures.
Conclusion
Merging properties of two JavaScript objects can be handled in a variety of ways depending on the specific requirements and object complexity. For simple, shallow merges, using the spread operator or Object.assign()
provide quick solutions. However, for more complex scenarios involving nested objects and the need to truly integrate properties, consider implementing a custom deep merge function. Apply these methods wisely to enhance your project’s data handling capabilities, ensuring efficient and effective data integration and manipulation.
No comments yet.