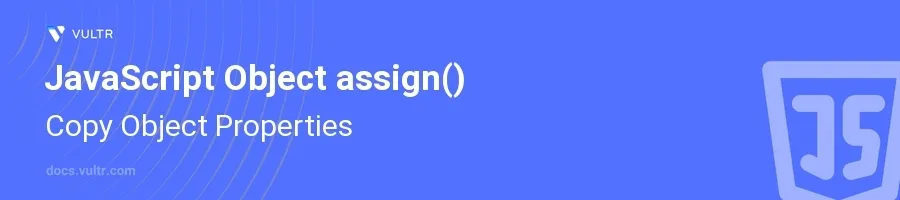
Introduction
The Object.assign()
method in JavaScript is integral for copying properties from one or more source objects to a target object. This method accomplishes shallow copies efficiently, making it a valuable tool in the Javascript programmer's toolkit, particularly when dealing with object manipulation and state management.
In this article, you will learn how to use the Object.assign()
method effectively. Explore techniques to copy properties between objects, merge objects, and address issues that arise with shallow copying, enhancing your ability to manage and manipulate object data in JavaScript.
Using Object.assign() to Copy Properties
Basic Copying from Source to Target
Define a target object where properties will be copied.
Define one or more source objects containing properties to copy.
Use
Object.assign()
to copy properties from the source objects to the target object.javascriptconst target = { a: 1 }; const source = { b: 2, c: 3 }; Object.assign(target, source); console.log(target);
This code copies all properties from the
source
object to thetarget
object, resulting in thetarget
object now having propertiesa
,b
, andc
.
Merging Multiple Objects
Start with a target object to merge properties into.
Define multiple source objects with distinct or overlapping properties.
Use
Object.assign()
to merge all source objects into the target.javascriptconst target = { a: 1 }; const source1 = { b: 2 }; const source2 = { c: 3, a: 4 }; Object.assign(target, source1, source2); console.log(target);
This example demonstrates merging properties from two sources into the target. It illustrates that properties from later sources overwrite those from earlier sources if they have the same keys, as seen with the property
a
.
Addressing Shallow Copy Limitations
Understanding the Shallow Copy
Recognize that
Object.assign()
performs a shallow copy, not a deep copy. This means nested objects are not cloned but referenced.Observe how this behavior affects the integrity of copied properties if the source object contains nested objects.
javascriptconst target = {}; const source = { a: { b: 2 } }; Object.assign(target, source); source.a.b = 3; console.log(target.a.b); // Outputs 3
In this scenario, changing a property in the original object also affects the copied object since the nested object was copied by reference.
Strategies to Handle Nested Objects
For a deep copy, consider alternative methods when dealing with nested objects.
Serialize and deserialize objects using JSON, or use other libraries like Lodash for deep copying.
javascriptconst deepCopy = function(source) { return JSON.parse(JSON.stringify(source)); }; const original = { a: { b: 2 } }; const target = {}; const source = deepCopy(original); Object.assign(target, source); original.a.b = 3; console.log(target.a.b); // Outputs 2
This technique involves creating a deep copy of the source object so that changes to the original object do not affect the copy. It's useful when you can't use libraries but is limited to JSON-serializable properties.
Conclusion
Using the Object.assign()
method in JavaScript allows for effective manipulation of objects, from copying properties between objects to merging them. Being aware of its shallow copy nature ensures that you apply correct techniques when handling more complex, nested objects. By mastering Object.assign()
and understanding its limitations, you can enhance functionality and robustness in your JavaScript applications, dealing with objects cleanly and efficiently.
No comments yet.