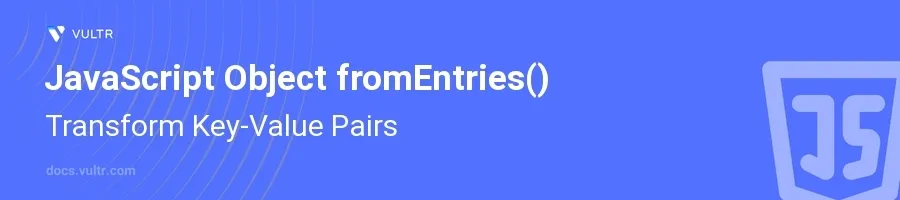
Introduction
The fromEntries()
method in JavaScript provides a robust solution for transforming key-value pairs into an object. This method is particularly useful when manipulating data received from various sources, like APIs or handling transformations within data structures. It makes the conversion of arrays or other iterable objects containing key-value pairs into a standard object straightforward and clean.
In this article, you will learn how to use the fromEntries()
method in JavaScript effectively in various data manipulation scenarios. Explore the mechanics of this method, see how it simplifies the data transformation process, and understand its integration with other JavaScript functionalities to manage data more efficiently.
Using fromEntries() in JavaScript in Different Scenarios
Using fromEntries() to Convert an Array of Arrays into an Object
Prepare an array of arrays where each inner array consists of two elements representing a key and a value respectively.
Use the
fromEntries()
method to convert this array into an object.javascriptconst entries = [['name', 'Alice'], ['age', 25]]; const obj = Object.fromEntries(entries); console.log(obj);
This code snippet converts the
entries
array into an object where 'name' and 'age' become the keys, associated with their respective values, 'Alice' and 25.
Using fromEntries() to Convert a Map Data Structure into an Object
Utilize the
Map
object to store data as key-value pairs.Convert the
Map
to an object usingfromEntries()
.javascriptconst map = new Map([['fruit', 'apple'], ['color', 'red']]); const objFromMap = Object.fromEntries(map); console.log(objFromMap);
Here, a
Map
containing the pairs 'fruit'-'apple' and 'color'-'red' is converted into a regular object. This transformation allows for the further manipulation of map data using standard object operations.
Working with Dynamic Data Retrieval
Fetch data dynamically, for example, querying a database or an API that returns data as key-value pairs in array format.
Convert this dynamically fetched data into objects for easier manipulation.
javascriptasync function fetchData() { const response = await fetch('https://api.example.com/data'); const data = await response.json(); // assuming data comes as an array of arrays const obj = Object.fromEntries(data); console.log(obj); } fetchData();
In this code, data fetched from a hypothetical API is transformed into an object using
fromEntries()
, facilitating easier access and manipulation of each data item based on its key.
Conclusion
Using the fromEntries()
method in JavaScript significantly simplifies the transformation of iterable key-value pairs into objects. This functionality is invaluable when dealing with data processing tasks that require conversion from structures like arrays or maps into more manageable JavaScript objects. By mastering fromEntries()
, you enhance your ability to handle various data transformation scenarios effectively, making your JavaScript code cleaner and more efficient.
No comments yet.