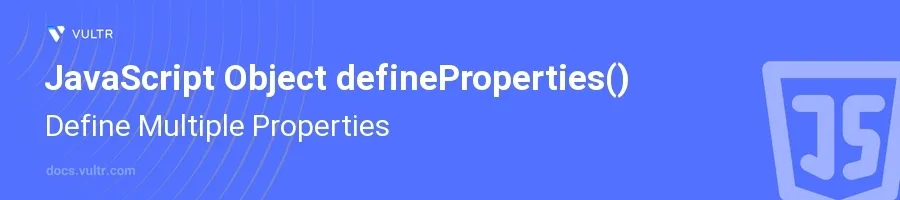
Introduction
The Object.defineProperties()
method in JavaScript is a powerful feature that allows developers to define multiple properties on an object at once while specifying attributes for each property, such as whether they are writable, enumerable, or configurable. This method provides finer control over properties compared to traditional methods of adding properties to objects.
In this article, you will learn how to effectively use the Object.defineProperties()
method. Explore how to define multiple properties with various configurations on JavaScript objects and understand the impact of different attributes on these properties.
Using Object.defineProperties()
Basics of Object.defineProperties()
Understand the syntax and parameters of
Object.defineProperties()
. The method takes two parameters: the object on which properties should be defined and an object that describes the properties.javascriptObject.defineProperties(obj, props);
obj
: The object on which properties will be defined.props
: An object whose keys represent property names and values are descriptors describing the configuration of the property.
Defining Multiple Properties
Start by creating an object on which you want to define properties.
Use
Object.defineProperties()
to add multiple properties with their descriptors.javascriptconst book = {}; Object.defineProperties(book, { title: { value: 'JavaScript: The Good Parts', writable: true, configurable: true, enumerable: true }, author: { value: 'Douglas Crockford', writable: true, configurable: true, enumerable: true }, year: { value: 2008, writable: false, configurable: true, enumerable: true } });
This code defines three properties on the
book
object. Theyear
property is set as non-writable, meaning its value can't be changed after its initial definition.
Attribute Impact on Properties
Explore how the attributes
writable
,enumerable
, andconfigurable
affect property behavior.writable
: Determines if the property value can be changed.enumerable
: Determines if the property appears infor...in
loops andObject.keys()
.configurable
: Determines if the property can be removed from the object and whether its attributes (except forwritable
) can be changed.
Experiment with these attributes to understand their effects on properties and property enumeration.
Use Cases in Real Applications
Apply
Object.defineProperties()
in practical scenarios such as creating immutable objects. For instance, you might want to protect configuration settings from being changed at runtime.javascriptconst configSettings = {}; Object.defineProperties(configSettings, { apiUrl: { value: "https://api.example.com", writable: false, // Prevent modification enumerable: true, configurable: false // Prevent deletion and reconfiguration }, maxConnections: { value: 100, writable: false, enumerable: true, configurable: false } });
In this snippet, the properties of
configSettings
are set as non-writable and non-configurable, ensuring they remain unchanged and undeletable throughout the application lifecycle.
Conclusion
Object.defineProperties()
offers a robust way to define multiple properties on JavaScript objects while providing detailed control over property configurations. By utilizing this method, you can enhance the integrity and manageability of properties in your JavaScript applications, ensuring that objects behave exactly as intended under various conditions. Familiarize yourself with the attributes and use cases discussed to maximize the benefits of this method in your JavaScript development tasks.
No comments yet.