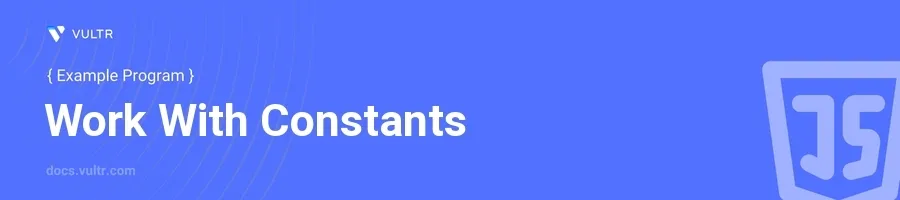
Introduction
Constants in JavaScript are fixed values that, once assigned, should not change during the execution of a script. Defined using the const
keyword, constants are block-scoped, much like variables defined using let
, but with the added feature that the value they hold cannot be reassigned. This brings an additional layer of security to your code by preventing accidental value changes which might be difficult to debug.
In this article, you will learn how to harness the power of constants in JavaScript through practical examples. Explore different use cases such as defining basic constants, creating constant objects, and working with constants in functions. The examples provided will guide you in using constants effectively to make your programs more reliable and maintainable.
Understanding Basic Constants
Define a Simple Constant
Declaring a constant for primitive data types like strings or numbers:
javascriptconst PI = 3.14159; console.log(PI);
This snippet demonstrates defining
PI
as a constant, which holds the value3.14159
. Note that attempting to reassign a value toPI
will result in a runtime error.
Attempting to Modify a Constant
Observe what happens when trying to reassign a constant:
javascriptconst GREETING = "Hello, world!"; GREETING = "Hello, again!"; // This line will cause an error
In this code, the attempt to reassign
GREETING
will throw a TypeError because constants cannot be reassigned after their initial declaration.
Working with Constant Objects
Define an Object as a Constant
Creating a constant object but modifying its properties:
javascriptconst CAR = { brand: "Toyota", model: "Corolla" }; CAR.model = "Camry"; console.log(CAR);
Here, the
CAR
object is defined as a constant, but itsmodel
property can be changed. This highlights an important aspect of constant objects: while the object itself cannot be reassigned, its properties can still be altered.
Adding Properties to a Constant Object
Adding new properties to the constant object:
javascriptCAR.year = 2021; console.log(CAR);
This code adds a new property
year
to theCAR
object. Such modifications are permitted because the reference to the object hasn’t changed, only the content has.
Constants in Functions
Utilizing Constants Inside Functions
Define constants within a function scope:
javascriptfunction calculateArea(radius) { const PI = 3.14159; const area = PI * radius * radius; return area; } console.log(calculateArea(10));
This function calculates the area of a circle with a given radius. The constant
PI
is safely encapsulated within thecalculateArea
function, showcasing another use case whereconst
helps maintain the integrity of the values within a specific scope.
Conclusion
Constants play a crucial role in JavaScript programming by ensuring that values that are meant to remain unchanged do so throughout the script’s execution. Whether you're working with basic data types, objects, or within functions, constants can help prevent bugs and make your code more predictable and easier to understand. By integrating the techniques discussed, you enhance the robustness and security of your applications, making them less prone to errors arising from inadvertent data modifications.
No comments yet.