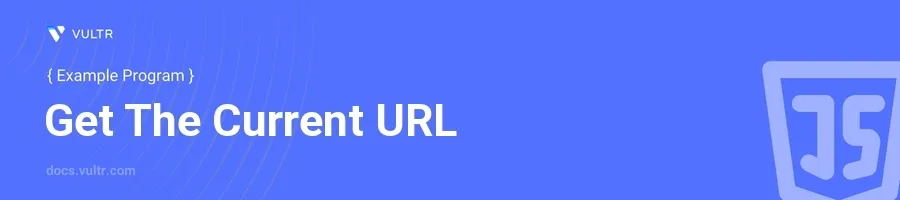
Introduction
The ability to retrieve the current URL in JavaScript is a ubiquitous requirement in modern web development, ranging from redirect functionalities to dynamic content loading. Accessing the URL allows developers to extract specific parameters, paths, or query strings, which can influence the behavior of web applications.
In this article, you will learn how to use JavaScript to get the current URL in various forms. The discussions include obtaining the full URL, separate components like the protocol, hostname, and path, and how to work with URL query parameters effectively.
Retrieving the Full URL
Get the Complete URL of the Current Page
Access the
window.location.href
property to retrieve the entire URL of the current page.javascriptlet currentUrl = window.location.href; console.log(currentUrl);
This code snippet will display the complete URL of the current page in the console. The
window.location.href
contains all parts of the URL including protocol, hostname, port (if specified), pathname, query string, and hash.
Extracting URL Components
Access Protocol, Host, and Pathname
Use properties of
window.location
to get individual URL components such as protocol, host, and pathname.javascriptlet protocol = window.location.protocol; let host = window.location.host; let pathname = window.location.pathname; console.log(`Protocol: ${protocol}`); console.log(`Host: ${host}`); console.log(`Pathname: ${pathname}`);
Here,
protocol
will show the protocol used (e.g.,http:
orhttps:
),host
provides the hostname and port (if present), andpathname
displays the path component of the URL.
Extracting the Query String
Retrieve the query string from the URL using
window.location.search
.javascriptlet queryString = window.location.search; console.log(queryString);
This line fetches the query string part of the URL, which starts with
?
and consists of key-value pairs. It's particularly useful for accessing data passed as parameters in GET requests.
Working with Query Parameters
Parse Query Parameters into an Object
Create a function to convert the query string into a more accessible object format.
javascriptfunction getQueryParams(queryString) { let params = new URLSearchParams(queryString); let obj = {}; params.forEach((value, key) => { obj[key] = value; }); return obj; } let queryParams = getQueryParams(window.location.search); console.log(queryParams);
This function uses
URLSearchParams
, a built-in web API for working with the query string, to parse it and convert the parameters into a JavaScript object. It provides an easy way of accessing parameter values using keys.
Conclusion
Retrieving and manipulating the URL and its components in JavaScript is straightforward yet powerful for controlling the flow and functionality of web applications. Understanding how to access the full URL, decompose it into its basic components, and manipulate query strings enables precise control over content and user experience based on URL parameters. By applying the techniques discussed, you ensure your applications can dynamically respond to changes in URL and extract necessary data efficiently.
No comments yet.