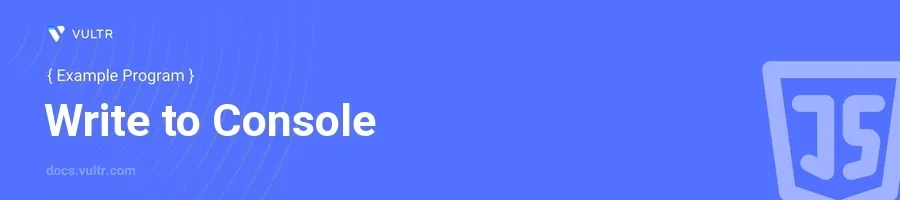
Introduction
JavaScript offers various methods to write or log information to the console, an invaluable feature during the development process for debugging and data inspection. Whether you're working with simple data or complex objects, understanding how to effectively utilize console methods is critical in JavaScript development.
In this article, you will learn how to use different JavaScript methods to write to the console. Discover when and why to use specific console methods like console.log()
, console.error()
, console.warn()
, and more through practical examples.
Basic Console Logging
Using console.log
Begin by using the most common method,
console.log()
, which outputs general messages or variables to the browser's JavaScript console.To demonstrate, write a simple string and a variable.
javascriptconst message = "Hello, console!"; console.log("Simple Message:", message);
This snippet prints a welcome message string concatenated with the variable
message
to the console.
Logging Multiple Variables
Utilize
console.log()
to print multiple values at once, which helps in debugging by allowing comparison of values side-by-side.Print several different data types together.
javascriptlet number = 30; let isActive = true; console.log("Number:", number, "Active:", isActive);
This code outputs the values of
number
andisActive
directly in the console, providing clear visibility of each variable's value.
Advanced Logging
Using console.table()
Employ
console.table()
to display data in a tabular format, ideal for arrays or objects.Log an array of objects to observe how
console.table()
represents it neatly.javascriptconst users = [ { name: "Alice", age: 25 }, { name: "Bob", age: 30 } ]; console.table(users);
In this example,
console.table()
effectively organizes theusers
array by showing indices and property values in a table format, making complex data easier to analyze.
Debugging with console.error()
Use
console.error()
to output error messages, making them stand out in the console with an error icon and typically red text.Simulate an error message.
javascriptconsole.error("An error occurred!");
This prints the error message
"An error occurred!"
distinctly in the console, highlighting it for developers.
Warnings with console.warn()
Invoke
console.warn()
to display warning messages that are less severe than errors but still require attention.Demonstrate by logging a simple warning.
javascriptconsole.warn("Warning: This is a warning message!");
This logs a warning to the console, which is typically displayed with a yellow color to differentiate it from standard logs and errors.
Special Console Features
Clearing the Console
Use
console.clear()
to clear the console window, useful in removing clutter during extensive debugging sessions.Just call it without any parameters.
javascriptconsole.clear();
Executing this method clears all previous console output, providing a fresh start for new logs.
Custom Console Styles
Enhance
console.log()
by adding custom CSS styles to make certain logs standout.Apply CSS directly within
console.log()
through string templates.javascriptconsole.log("%cThis message is styled!", "color: blue; font-size: large;");
This snippet demonstrates styling console output. The
%c
withinconsole.log()
is used to apply CSS styles, here changing the text color and font size.
Conclusion
Mastering console methods in JavaScript enhances your debugging skills and improves your code maintenance capabilities. From basic logging with console.log()
to more specialized methods like console.error()
and console.warn()
, the console object provides a suite of tools for different debugging scenarios. Implement these practices to refine your development process, making debug outputs more informative and visually distinct. Whether dealing with simple values or complex datasets, these console methods offer the flexibility and power needed for effective JavaScript development.
No comments yet.