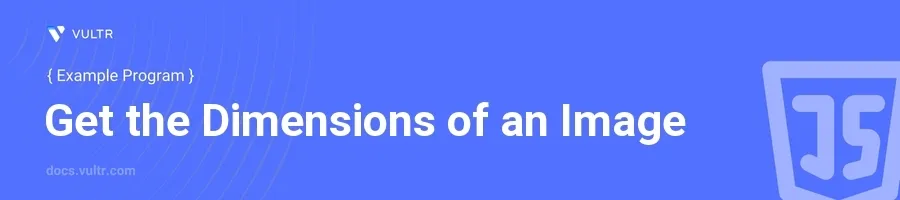
Introduction
Using JavaScript to obtain the dimensions of an image is a fundamental task that plays a critical role in various web development scenarios. Whether managing media galleries, validating image uploads, or optimizing responsive designs, retrieving image dimensions helps tailor applications to function smoothly across different devices and orientations.
In this article, you will learn how to leverage JavaScript to fetch the dimensions of an image in different contexts. You will explore multiple examples including loading images from the local file system and the web, ensuring you can apply these techniques effectively in your projects.
Accessing Image Dimensions Locally
Load and Measure Image from Local Source
Create an HTML file and integrate a JavaScript script to process images.
Use the HTML file input for selecting an image.
Write JavaScript to load the image and alert its dimensions after loading.
html<!DOCTYPE html> <html> <head> <title>Get Image Dimensions</title> </head> <body> <input type="file" id="imageInput" accept="image/*"> <script> const input = document.getElementById('imageInput'); input.addEventListener('change', function(event) { const file = event.target.files[0]; const img = new Image(); img.onload = function() { alert(`Width: ${img.width}, Height: ${img.height}`); }; img.src = URL.createObjectURL(file); }); </script> </body> </html>
This HTML and JavaScript combination prompts the user to select an image from their local device, and upon loading, displays an alert with the image dimensions.
URL.createObjectURL
creates a temporary URL from the file input, which the image element uses to load.
Accessing Image Dimensions from the Web
Fetch Image from a URL and Display Dimensions
Write an HTML structure with JavaScript that loads a specified image URL.
Use the
Image
object to load the image and get its dimensions once loaded.html<!DOCTYPE html> <html> <head> <title>Get Image Dimensions from URL</title> </head> <body> <script> const img = new Image(); img.onload = function() { alert(`Width: ${img.width}, Height: ${img.height}`); }; img.src = 'https://example.com/path/to/your/image.jpg'; </script> </body> </html>
In this script, an image URL is assigned to the
src
property of a newImage
object. When the image loads, the dimensions are retrieved and displayed using an alert. Ensure that the URL is accessible and allows cross-origin requests to avoid security errors.
Handling CORS Issues When Loading Images
Same-Origin Policy and Image Loading
Understand that loading images from different domains can lead to CORS (Cross-Origin Resource Sharing) restrictions.
Ensure the server hosting the image supports CORS if fetching from a different origin.
javascriptconst img = new Image(); img.crossOrigin = "Anonymous"; // Requesting CORS permission img.onload = function() { try { alert(`Width: ${img.width}, Height: ${img.height}`); } catch (e) { alert('Failed to load image due to CORS policies'); } }; img.src = 'https://example.com/path/to/your/image.jpg';
Setting the
crossOrigin
attribute to "Anonymous" on theImage
object requests the server to provide CORS approval. This way, the image can be loaded without restrictions if the server is configured correctly.
Conclusion
Accessing the dimensions of an image using JavaScript is an indispensable skill in web development. Through the various approaches detailed here, mastering image manipulation in both local and web contexts is attainable. Remember that handling images with respect to CORS requires proper server configuration. Apply these techniques to enhance your web applications by integrating dynamic and responsive image handling capabilities, ensuring a smooth user experience across different platforms and devices.
No comments yet.