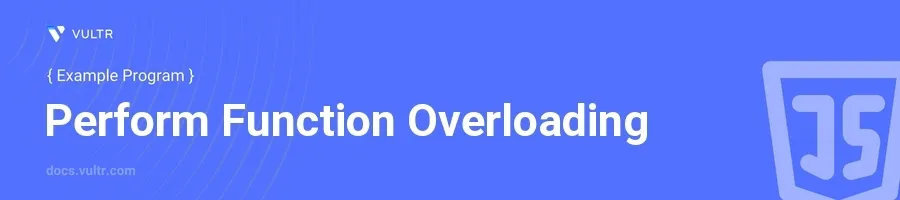
Introduction
Function overloading, a common concept in many programming languages, facilitates the creation of multiple functions with the same name but different parameters. However, JavaScript does not natively support function overloading in the traditional sense found in languages like Java or C#. Instead, JavaScript developers must creatively achieve similar functionality through flexible parameter handling and type checking within functions.
In this article, you will learn how to simulate function overloading in JavaScript. Explore different techniques such as using optional parameters, arguments object, and explicit checks within a single function to handle various input scenarios. This approach empowers developers to craft functions that are adaptable to multiple use cases, enhancing their applications' flexibility.
Simulating Function Overloading in JavaScript
Using Optional Parameters
Define a function that accepts a set number of optional parameters using default values.
Adjust the function's behavior based on which parameters are provided.
javascriptfunction multiply(a, b = 1) { return a * b; } console.log(multiply(5)); // Outputs: 5 console.log(multiply(5, 2)); // Outputs: 10
Here, the
multiply
function can perform different behaviors: When only one argument is provided, it assumes the second argument as1
. When both are provided, it multiplies both. This technique allows for handling different numbers of parameters without generating multiple function definitions.
Utilizing the Arguments Object
Create a function that doesn't specify any fixed parameters.
Use the
arguments
object provided by JavaScript to handle any number of parameters.javascriptfunction addNumbers() { let result = 0; for (let i = 0; i < arguments.length; i++) { result += arguments[i]; } return result; } console.log(addNumbers(3, 4, 5)); // Outputs: 12 console.log(addNumbers(3, 4)); // Outputs: 7
In this example,
addNumbers
can sum an arbitrary number of numbers, demonstrating a form of overloading. Since the function doesn't restrict the number of arguments, it intelligently processes whichever arguments it receives.
Explicit Parameter Checks
Craft a single function that explicitly checks the number and type of arguments it receives, altering its behavior accordingly.
Implement logical branches inside the function to treat different scenarios.
javascriptfunction mixArgs(first, second) { if (arguments.length === 1 && typeof first === 'string') { return `String detected: ${first}`; } else if (arguments.length === 2 && typeof first === 'number' && typeof second === 'number') { return `Sum of numbers: ${first + second}`; } return `Default implementation`; } console.log(mixArgs('Hello!')); // Outputs: String detected: Hello! console.log(mixArgs(5, 10)); // Outputs: Sum of numbers: 15
The function
mixArgs
differentiates its execution based on the count and type of arguments it processes, mirroring traditional overloading mechanisms. This method offers a robust solution for handling different types of inputs, ensuring the function is adaptable for various data types and operations.
Conclusion
Simulating function overloading in JavaScript involves creative use of the language's flexible handling of function parameters. Techniques such as using default parameters, the arguments
object, and conditional checks within a function enable you to manage multiple input scenarios effectively. By implementing these strategies, enhance your JavaScript functions' adaptability, making them capable of handling a diversity of tasks with a single functional declaration. Embrace these practices to make your JavaScript code more robust, maintainable, and efficient.
No comments yet.