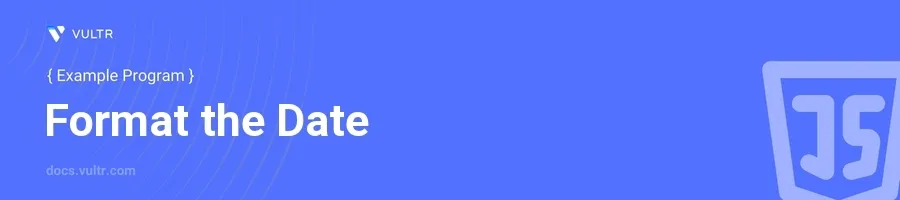
Introduction
Handling dates and formatting them according to specific requirements is a common task in web development. JavaScript offers various methods to manipulate and format dates, which can be quite handy in a myriad of applications, from displaying date stamps on posts to handling time-sensitive transactions.
In this article, you will learn how to format dates using JavaScript. You will explore different ways to display dates as per common requirements, such as changing the date format, displaying only parts of the date, and even handling time zones. Practical examples will help you understand these concepts clearly.
Basic Date Formatting in JavaScript
Display the Current Date in YYYY-MM-DD Format
Instantiate a new
Date
object to get the current date.Extract the year, month, and day using the
getFullYear()
,getMonth()
, andgetDate()
methods.Format the month and day to ensure they appear as two digits.
javascriptconst currentDate = new Date(); const formattedDate = `${currentDate.getFullYear()}-${(currentDate.getMonth() + 1).toString().padStart(2, '0')}-${currentDate.getDate().toString().padStart(2, '0')}`; console.log(formattedDate);
This example creates a formatted string in "YYYY-MM-DD" format. The month and day are padded with zeros if necessary to maintain a two-digit format, using
padStart()
method.
Formatting a Date Object into a Readable String
Use the
Date
object'stoDateString()
method to convert a date into a more readable form.javascriptconst date = new Date(); const readableDate = date.toDateString(); console.log(readableDate);
The
toDateString()
method returns a date as a human-readable string, omitting the time portion.
Advanced Date Formatting Using Locale
Display Date in a Locale-Sensitive Format
Utilize the
toLocaleDateString()
method of theDate
object to format the date according to a specific locale.Pass locale and options to the method to customize the output.
javascriptconst eventDate = new Date(); const locale = 'en-US'; const options = { weekday: 'long', year: 'numeric', month: 'long', day: 'numeric' }; const localizedDate = eventDate.toLocaleDateString(locale, options); console.log(localizedDate);
This snippet formats the
eventDate
according to the specified options and locale, allowing for a region-specific display of the date.
Using Multiple Locales
Format a date using multiple locales to see differences in date representation.
javascriptconst someDate = new Date(); const locales = ['en-US', 'de-DE', 'ja-JP']; locales.forEach(locale => { console.log(someDate.toLocaleDateString(locale)); });
Here,
toLocaleDateString()
is called with different locales to demonstrate how the date appears in American English, German, and Japanese.
Handling Time Zones in Date Formatting
Display Date and Time with Time Zone Information
Adjust the
toLocaleString()
method parameters to handle different time zones.javascriptconst now = new Date(); const timeZone = 'Asia/Tokyo'; const timeZoneOptions = { timeZoneName: 'long' }; console.log(now.toLocaleString('en-US', { ...timeZoneOptions, timeZone }));
In this example, the date and time are displayed along with the full name of the time zone, demonstrating how to manipulate time zone data in date formatting.
Conclusion
JavaScript provides various methods to format and manipulate dates, adaptable to almost any development scenario. From simple date displays to complex locale and time zone handling, the methods discussed here enable robust date formatting. Whether for user interfaces, logs, or data storage, mastering these tools ensures that date-related data is both human-readable and precise. Use the examples provided as a basis to expand further into JavaScript's date-handling capabilities, adapting and modifying them as your projects require.
No comments yet.