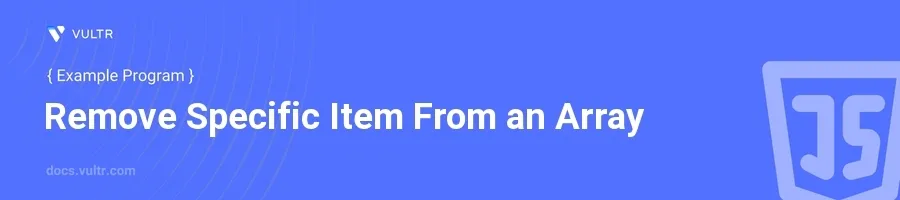
Introduction
Removing specific items from an array is a fundamental operation in JavaScript programming, especially when managing lists of elements such as user data, settings, or inputs for further operations. Being able to manipulate arrays efficiently allows programmers to handle data more robustly and tailor user interactions more dynamically.
In this article, you will learn how to effectively remove specific items from an array in JavaScript. Explore various methods and scenarios where each technique is advantageous, including using high-level array manipulation functions and understanding the resulting implications for the array's structure and the performance of your code.
Identify and Remove Using Splice
Basic Removal by Index
Determine the index of the item to be removed.
Use the
splice()
method to remove the item.javascriptlet fruits = ['apple', 'banana', 'orange']; let indexToRemove = 1; // Index of 'banana' fruits.splice(indexToRemove, 1); console.log(fruits);
This code removes 'banana' from the
fruits
array. Thesplice()
method is versatile as it can both remove and add elements at any specific index, making it particularly useful for direct index manipulations.
Removing Multiple Items
Use the
splice()
method specifying the start index and the number of items to remove.javascriptlet colors = ['red', 'green', 'blue', 'yellow', 'purple']; colors.splice(1, 3); // removes 'green', 'blue', 'yellow' console.log(colors);
Here,
colors.splice(1, 3)
starts at index 1 ('green') and removes three items including 'green', 'blue', and 'yellow'.
Filter Out Specific Values
Using the filter()
Method
Utilize
filter()
to create a new array excluding the unwanted items.Define the condition to retain elements.
javascriptlet numbers = [1, 2, 3, 4, 5]; let filteredNumbers = numbers.filter(number => number !== 3); console.log(filteredNumbers);
The
filter()
method creates a new array calledfilteredNumbers
that includes all elements except for3
. This method avoids modifying the original array and is ideal for cases where immutability of the original data is crucial.
Conditionally Removing Objects from Array of Objects
Create a condition that matches certain properties of objects.
javascriptlet users = [ { id: 1, name: 'John' }, { id: 2, name: 'Jane' }, { id: 3, name: 'Joe' } ]; let activeUsers = users.filter(user => user.id !== 2); console.log(activeUsers);
In this snippet, all user objects except for the one with
id: 2
are included inactiveUsers
. This technique is useful for filtering data based on complex conditions or multiple properties.
Using the findIndex
and splice
Combination
Removing an Item by Property
Find the index using
findIndex()
.Check the found index and apply
splice()
if valid.javascriptlet people = [ { id: 10, name: 'Alice' }, { id: 20, name: 'Bob' }, { id: 30, name: 'Charlie' } ]; let index = people.findIndex(person => person.id === 20); if (index !== -1) { people.splice(index, 1); } console.log(people);
This approach first finds the index of the item with
id: 20
and then removes it usingsplice
. Handling the index check before splicing prevents possible errors from trying to remove items that don't exist.
Conclusion
Mastering different techniques to remove specific items from an array in JavaScript enhances your ability to manipulate and manage data within your applications. Whether updating the existing array directly or creating a new array to hold filtered results, choose the method that best suits the scenario based on performance considerations and the need for data integrity. Equip yourself with these skills to write cleaner, more efficient JavaScript code.
No comments yet.