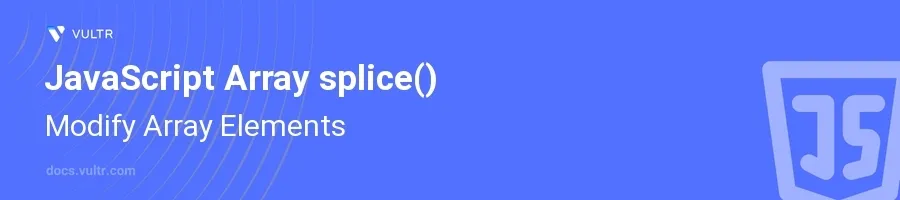
Introduction
The splice()
method in JavaScript is a versatile tool used to modify array elements by adding, removing, or replacing them. This powerful array method can directly alter the content of the array and is beneficial for managing data in dynamic applications where array manipulation is frequently required.
In this article, you will learn how to use the splice()
method in various scenarios. Explore the syntax of splice()
and how to efficiently add, remove, and replace elements in arrays, enhancing your ability to handle array data effectively.
Understanding the splice() Method
Basic Syntax of splice()
- Recognize the parameters of the
splice()
method:start
: The index at which to start changing the array.deleteCount
: The number of elements to remove from the array.item1, item2, ...
: The elements to add to the array.
Syntax Example
Look at the following basic usage of
splice()
.javascriptlet myArray = ['a', 'b', 'c', 'd']; myArray.splice(1, 2, 'x', 'y'); console.log(myArray);
This code modifies
myArray
by removing two elements starting from index1
and adds 'x' and 'y' in their place.
Adding Elements with splice()
Insert Without Removing
Understand how to insert elements without removing any existing elements.
Use
splice()
withdeleteCount
set to0
.javascriptlet numbers = [1, 2, 4, 5]; numbers.splice(2, 0, 3); console.log(numbers);
Here,
3
is inserted into the arraynumbers
at index2
without removing any elements, effectively shifting the other elements to higher indices.
Removing Elements with splice()
Simple Removal
Learn to remove elements without adding new ones.
Set
deleteCount
and omit the addition parameters.javascriptlet fruits = ['apple', 'banana', 'orange', 'mango']; fruits.splice(1, 2); console.log(fruits);
This code removes 'banana' and 'orange' from the
fruits
array, demonstrating howsplice()
can be used to delete elements efficiently.
Replacing Elements with splice()
Element Replacement
Combine removal and addition to replace elements.
Use
splice()
to remove elements and add new ones at the same index.javascriptlet languages = ['JavaScript', 'Python', 'Java']; languages.splice(2, 1, 'TypeScript'); console.log(languages);
With this snippet, 'Java' is replaced by 'TypeScript' in the
languages
array. It shows howsplice()
handles both removal and addition in one operation.
Conclusion
The splice()
function in JavaScript is an essential tool for array manipulation, offering capabilities to add, remove, and replace elements simultaneously. Mastering this method allows for more dynamic data management in JavaScript applications, simplifying complex array transformations. By utilizing the examples and explanations provided, empower your JavaScript coding to efficiently manage and modify array data.
No comments yet.