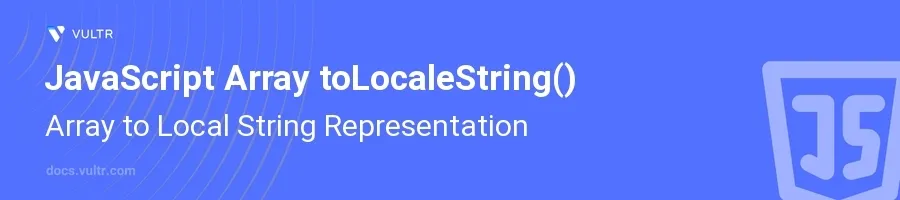
Introduction
The JavaScript method toLocaleString()
provides a way to convert elements of an array into a localized string representation. This function is particularly useful when working with data that needs to be presented according to the cultural norms and formatting styles of a user’s locale, such as dates, times, or numbers.
In this article, you will learn how to leverage the toLocaleString()
method to convert array elements based on local settings. Explore how to handle basic arrays, arrays containing date objects, and mixed-type arrays, and see how different options can affect the output of this versatile method.
Basic Usage of toLocaleString()
Convert Numeric Arrays
Create an array of numbers.
Apply the
toLocaleString()
method to convert the array into a localized string format.javascriptconst numbers = [1234, 5678, 9012]; const localizedNumbers = numbers.toLocaleString(); console.log(localizedNumbers);
In this example,
toLocaleString()
converts each number in the array into a string formatted according to default locale settings, typically resulting in comma-separated values.
Localizing with Specific Locale
Specify a locale to customize the formatting further.
Convert the numbers using the specified locale settings.
javascriptconst numbers = [1234, 5678, 9012]; const localizedGerman = numbers.toLocaleString('de-DE'); console.log(localizedGerman);
Here, the numbers are formatted using German locale settings, resulting in a period as the thousand separator.
Handling Arrays with Date Objects
Localize Dates in an Array
Create an array containing date objects.
Use
toLocaleString()
to convert each date to a locale-specific string.javascriptconst dates = [new Date(), new Date()]; const localizedDates = dates.toLocaleString('en-US', { weekday: 'long', year: 'numeric', month: 'long', day: 'numeric' }); console.log(localizedDates);
Each date in the array is converted into a more readable, localized format including full weekday, year, month, and day names based on the 'en-US' locale. Customization options such as formatting styles can be specified in the options object.
Mixed Data Types in Arrays
Converting Mixed Type Arrays
Prepare an array containing mixed types such as strings, numbers, and dates.
Apply
toLocaleString()
to format each element based on its type.javascriptconst mixedArray = [new Date(), "Hello", 1234]; const localizedMixed = mixedArray.toLocaleString('fr-FR'); console.log(localizedMixed);
In this code snippet, the date is formatted according to French standards, strings remain unaffected, and numbers are formatted with locale-specific settings.
Conclusion
The toLocaleString()
method in JavaScript is an effective tool for converting array elements into a string representation that respects local conventions. Whether dealing with numbers, dates, or mixed-type arrays, this method offers flexibility to present data in a format that is familiar and readable to users based on their own cultural context. By mastering toLocaleString()
, incorporate localized data presentation into your JavaScript applications effortlessly.
No comments yet.