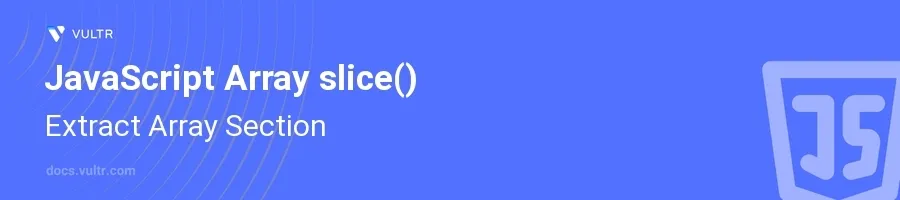
Introduction
The slice()
method in JavaScript is a powerful utility for extracting a shallow copy of a portion of an array into a new array object. This method is essential when you need to work with subsets of array elements without altering the original array. It provides flexibility in handling array data, especially in scenarios involving non-destructive operations.
In this article, you will learn how to effectively use the slice()
method to manipulate array data in JavaScript. Explore how to extract specific sections of arrays using this method, understand its parameters, and see common use cases where slice()
proves invaluable.
Understanding the slice() Method
Syntax and Parameters
The slice()
method accepts two parameters:
- start (optional): The zero-based index at which to start extraction.
- end (optional): The zero-based index before which to end extraction. The element at this index is not included.
If start
is undefined, slice()
starts from the beginning of the array. If end
is omitted, slice()
extracts through the end of the sequence. Here’s a breakdown of its behavior:
- When both
start
andend
are positive,slice()
extracts from the start index up to, but not including, the end index. - If
start
is undefined, the extraction starts from index 0. - When
end
is undefined, the extraction goes through to the end of the array. - If
start
is greater than the length of the array, an empty array is returned.
Using slice() to Create a Subarray
Define an array containing various elements.
Use
slice()
to extract a part of the array from a specified start index to an end index.javascriptconst fruits = ['Apple', 'Banana', 'Cherry', 'Date']; const citrus = fruits.slice(1, 3); console.log(citrus);
In this example,
slice(1, 3)
extracts elements with indices 1 and 2 (‘Banana’ and ‘Cherry’) from thefruits
array. ‘Date’ is not included because the end index inslice()
is exclusive.
Handling Negative Indices
Understand that negative indices count backward from the end of the array.
Use negative indices with
slice()
to get elements from the end of the array.javascriptconst numbers = [10, 20, 30, 40, 50]; const lastTwo = numbers.slice(-2); console.log(lastTwo);
This code snippet extracts the last two elements of the
numbers
array, since-2
starts the slice two indices from the end.
Common Use Cases for slice()
Cloning an Array
Use
slice()
with no arguments to clone an entire array, which is a common operation to avoid mutations on the original array during manipulations.javascriptconst originalArray = [1, 2, 3, 4]; const clonedArray = originalArray.slice(); console.log(clonedArray);
This technique uses
slice()
to create a shallow copy oforiginalArray
. Changes inclonedArray
do not affectoriginalArray
unless mutable objects are involved.
Removing Elements Non-destructively
Combine
slice()
from different segments around an unwanted element or range and concatenate the results.Perform this operation when you need to simulate the removal of elements without using methods that alter the original array.
javascriptconst data = ['a', 'b', 'c', 'd', 'e']; const newData = data.slice(0, 2).concat(data.slice(3)); console.log(newData);
This code removes 'c' (at index 2) from
data
by extracting and concatenating the elements before and after 'c'.
Conclusion
The slice()
method in JavaScript is an invaluable tool for handling arrays, particularly when non-destructive techniques are required. By mastering slice()
, you gain greater flexibility and precision in data manipulation within your applications. Utilize this function to simplify array adjustments, make copies for safe transformations, or selectively extract portions of array data for processing. Utilize the examples and techniques discussed to enhance the efficiency and cleanliness of your JavaScript code.
No comments yet.